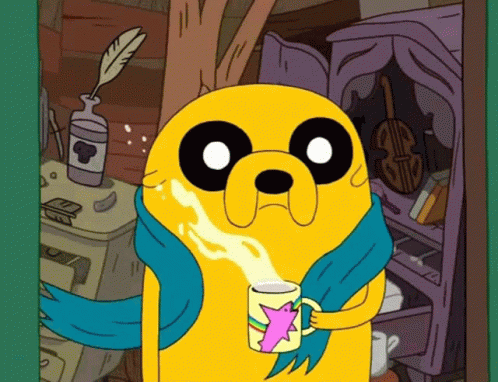
By
Braxy,
in Guides & HowTo
Similar Content
-
Similar Content
-
Similar Content
-
Tags
-
Activity
-
-
1420
[40250] Reference Serverfile + Client + Src [15 Available Languages]
Nice, thanks for sharing! -
9
GR2/DDS encryption
Don't think there's anything wrong specifically with this community. That's just how the world works If you're not open for critics then you should probably not make something public that you cannot defend... I understand that it's made for designers but you're giving them false hopes. -
0
Little problem with quest scrolls and quickslot
The following issue, this does not occur with every quest but with most of them: Due to the Quickslot system, the quests are shifted to the right, but for some quests, they shift back to the left + an inventory button is still visible. [Hidden Content] It's the same quest, but in another state there everything is normal. [Hidden Content] It would be kind if someone could tell me what the problem is and how it could be resolved. -
29
-
1
-
1420
[40250] Reference Serverfile + Client + Src [15 Available Languages]
The translation to mob_proto does not work for me, I did everything in the guide, but in Navicat it is still in a foreign language -
66
official Official Pickup Slot Effect [REVERSED]
Thank you, it actually solved the issue however it is not clear for me why the issue came from the quickslot's items. My 'fear' is there's still some issue and it's just a workaround to hide it. -
29
OLDGODSMT2 [ MEGA SF RELEASE ]
If the source archive is updated, the resources will be saved on metin2.download. Otherwise, this topic will have no backup, I no longer store VDI.
-
-
Recently Browsing
- No registered users viewing this page.
Recommended Posts