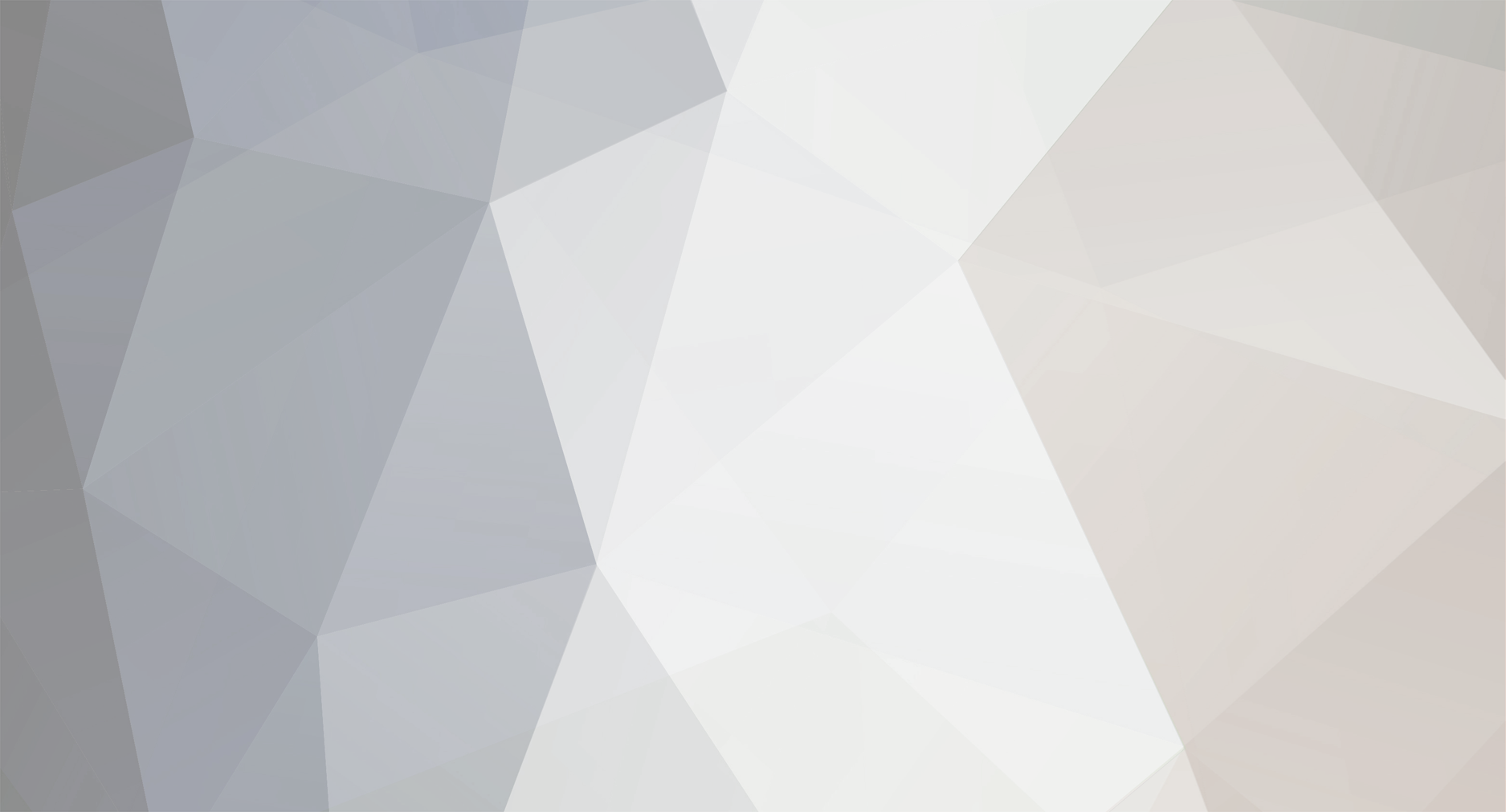
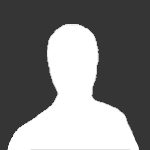
ridetpro
Banned-
Posts
106 -
Joined
-
Last visited
-
Days Won
1 -
Feedback
0%
Content Type
Forums
Store
Third Party - Providers Directory
Feature Plan
Release Notes
Docs
Events
Everything posted by ridetpro
-
It's probably not an error, it's a code problem. The client must be set to debug mode. Now change the launcher's output directory to the client's folder. Now make this change in Debugging. (UserInterface -> Properties -> Debugging) Open a new debugging instance: In this way, you will be able to see the call stack. Once the debug instance begins, log in to the client and wait for the crash. When the crash will appear you will be able to see callStack in visual studio ..
-
The compiler will choose the actual type used based on the enumeration constants. But you can do the following: // Enum can handle long long enum EFoo : std::int64_t { Foo1, Foo2, Foo3, } // Enum can handle int enum EFoo : std::int32_t { Foo1, Foo2, Foo3, }
-
[get_language()] in when ... begin
ridetpro replied to flexio's topic in Community Support - Questions & Answers
qc.cc parse() case ST_WHEN_NAME before current_when_argument += os.str(); I did something similar some time ago, I gave you the clues to look for. If you fail, I'll give you the codes. Follow the example above. -
Click: CQuestManager
ridetpro replied to Alexander2010's topic in Community Support - Questions & Answers
You need to add NPC vnum in questnpc.txt- 1 reply
-
- 1
-
-
Cannot load MOBDropItemFile
ridetpro replied to Mind Rapist's topic in Community Support - Questions & Answers
big words -
Linker command failed
ridetpro replied to Mind Rapist's topic in Community Support - Questions & Answers
Then you probably did not put the cryptopp7 headers in extern. -
Linker command failed
ridetpro replied to Mind Rapist's topic in Community Support - Questions & Answers
Compile cryptopp with c++17 too and with c++devel -
void CGuild::AddComment(LPCHARACTER ch, const std::string &str) { int player_id = ch->GetPlayerID(); if (str.length() > GUILD_COMMENT_MAX_LEN) { return; } char text[GUILD_COMMENT_MAX_LEN * 2 + 1]; DBManager::Instance().EscapeString(text, sizeof(text), str.c_str(), str.length()); DBManager::Instance().FuncAfterQuery( [this, player_id] {this->RefreshCommentForce(player_id); }, "INSERT INTO guild_comment(guild_id, name, notice, content, time) VALUES(%u, '%s', %d, '%s', NOW())", m_data.guild_id, ch->GetName(), (str[0] == '!') ? 1 : 0, text); } Use std::function instead of any_function
-
// Intro pc_mount function from questlua_pc.cpp // Under LPCHARACTER ch = CQuestManager::instance().GetCurrentCharacterPtr(); // Add int CalcLastMoveSec = (get_dword_time() - ch->GetLastMountTime()) / 1000 + 0.5; if (CalcLastMoveSec < 1) // Replace 1 with seconds number. { return 0; }
-
Help protos korean replacement
ridetpro replied to Shamsara's topic in Community Support - Questions & Answers
You can do that with microsoft Excel if you use txt protos or a query if you use sql. But you can ignore those koreean names. -
account problem (autoloot,safebox atc.)
ridetpro replied to pifanek's topic in Community Support - Questions & Answers
maybe freebsd time is wrong ? -
error when compiling.. help
ridetpro replied to Syriza's topic in Community Support - Questions & Answers
-lssl To LDFLAGS -
Poison given by monster crash server
ridetpro replied to Karbust's topic in Community Support - Questions & Answers
Pay someone to solve your shitty crash. bool CHARACTER::Damage(LPCHARACTER pAttacker, int dam, EDamageType type) // returns true if dead { if (DAMAGE_TYPE_MAGIC == type) { dam = (int)((float)dam * (100 + (pAttacker->GetPoint(POINT_MAGIC_ATT_BONUS_PER) + pAttacker->GetPoint(POINT_MELEE_MAGIC_ATT_BONUS_PER))) / 100.f + 0.5f); } // With bool CHARACTER::Damage(LPCHARACTER pAttacker, int dam, EDamageType type) { if (pAttacker == NULL) { sys_err("CHARACTER::Damage"); sys_err("pAttacker is null, check your shitty server"); return false; } if (DAMAGE_TYPE_MAGIC == type) { dam = (int)((float)dam * (100 + (pAttacker->GetPoint(POINT_MAGIC_ATT_BONUS_PER) + pAttacker->GetPoint(POINT_MELEE_MAGIC_ATT_BONUS_PER))) / 100.f + 0.5f); } -
Indexing MySQL-Querys ?
ridetpro replied to akaya's topic in Community Support - Questions & Answers
[Hidden Content] What Does Indexing Do? Indexing is the way to get an unordered table into an order that will maximize the query’s efficiency while searching. When a table is unindexed, the order of the rows will likely not be discernible by the query as optimized in anyway and your query will therefore have to search through the rows linearly. That is to say the queries will have to search through every row to find the rows matching the conditions. You can imagine this would take a while. Looking through every single row is not very efficient. For example, the table below represents a table in a fictional datasource, that is completely unordered. COMPANY_ID UNIT UNIT_COST 10 12 1.15 12 12 1.05 14 18 1.31 18 18 1.34 11 24 1.15 16 12 1.31 10 12 1.15 12 24 1.3 18 6 1.34 18 12 1.35 14 12 1.95 21 18 1.36 12 12 1.05 20 6 1.31 18 18 1.34 11 24 1.15 14 24 1.05 If we were to run the following query: SELECT company_id, units, unit_cost FROM index_test WHERE company_id = 18 The database would have to search through all 17 rows in the order they appear in the table, basically from top to bottom, one at a time. So to search for all of the potential instances of the company_id number 18, the database must look through the entire table for all appearances of 18 in the company_id column. This will only get more and more time consuming as the size of the table increases. As the sophistication of the data increases what could eventually happen is that a table with one billion rows is joined with another table with one billion rows and now instead of taking x amount of time, the query now has to search through twice the amount of rows costing twice the amount of time. You can see how this becomes problematic in our ever data saturated world. Tables increase in size and searching increases in execution time. Querying an unindexed table, if presented visually, would look like this: What indexing does is sets up the column you’re search conditions are on in a sorted order to assist in optimizing query performance. With an index on the company_id column the table would then, essentially, “look” like this: COMPANY_ID UNIT UNIT_COST 10 12 1.15 10 12 1.15 11 24 1.15 11 24 1.15 12 12 1.05 12 24 1.3 12 12 1.05 14 18 1.31 14 12 1.95 14 24 1.05 16 12 1.31 18 18 1.34 18 6 1.34 18 12 1.35 18 18 1.34 20 6 1.31 21 18 1.36 Now, the database can search for company_id number 18 and return all the requested columns for that row then move on to the next row. If the next row’s comapny_id number is also 18 then it will return the all the columns requested in the query. If the next row’s company_id is 20, the query knows to stop searching and the query will finish. How does Indexing Work? In reality the database table does not reorder itself every time the query conditions change in order to optimize the query performance, that would be unrealistic. In actuality what happens is the index causes the database to create a data structure. The data structure type is very likely a B-Tree. The advantages of the B-Tree are numerous, the main advantage for our purposes is that it is sortable. When the data structure is sorted in order it makes our search more efficient for the obvious reasons we pointed out above. When the index creates a data structure on a specific column it is important to note that no other column is stored in the data structure. Our data structure for the table above will only contain the the company_id numbers. Units and unit_costwill not be held in the data structure. How Does the Database Know What Other Fields in the Table to Return? Database indexes will also store pointers which are simply reference information for the location of the additional information in memory. Basically the index holds the company_id and that particular row’s home address on the memory disk. The index will actually look like this: COMPANY_ID POINTER 10 _123 10 _129 11 _127 11 _138 12 _124 12 _130 12 _135 14 _125 14 _131 14 _133 16 _128 18 _126 18 _131 18 _132 18 _137 20 _136 21 _134 With that index, the query can search for only the rows in the company_idcolumn that have 18 and then using the pointer can go into the table to find the specific row where that pointer lives. The query can then go into the table to retrieve the fields for the columns requested for the rows that meet the conditions. If the search were presented visually, it would look like this: To Re-Cap Indexing Adds a Data Structure with the Column that has the search conditions and a pointer The Pointer is the address on the memory disk of the row with the rest of the information The Data Structure Index is Sorted to optimize query efficiency The Query looks for the specific row in the index, the index refers to the pointer which will find the rest of the information. The Index reduces the number of rows the query has to search through from 17 to four.- 1 reply
-
- 1
-
-
Poison given by monster crash server
ridetpro replied to Karbust's topic in Community Support - Questions & Answers
It has nothing to do with monsters. The attacker is NULL, that is the character that tries to attack the monsters. I guess. -
Poison given by monster crash server
ridetpro replied to Karbust's topic in Community Support - Questions & Answers
if (type == DAMAGE_TYPE_POISON) { if (GetHP() - dam <= 0) dam = GetHP() - 1; } // char_battle.cpp // REPLACE if (pAttacker and (type == DAMAGE_TYPE_POISON)) { if (GetHP() - dam <= 0) dam = GetHP() - 1; } Try -
DOWNLOAD_LINK
-
Here you have a tool developed by @Sanii Watch this video: [Hidden Content] From here you can download the tool: [Hidden Content]
-
You'll need to install (or enable) the Socket PHP extension
-
Searching for a solution
ridetpro replied to MaJeStiC's topic in Community Support - Questions & Answers
#delete request , didnt understand the question, Not tested. -
Temple Ochao - Black Floor
ridetpro replied to Karbust's topic in Community Support - Questions & Answers
As I said, you probably did something wrong. The solution is not HeighScale. I, like you, use the files from the official client and I did not have such problems. Try to USE THIS FILES for server. And also use default settings intro client.- 14 replies
-
- 16
-
-
-
-
-
-
Temple Ochao - Black Floor
ridetpro replied to Karbust's topic in Community Support - Questions & Answers
Check again whole environment