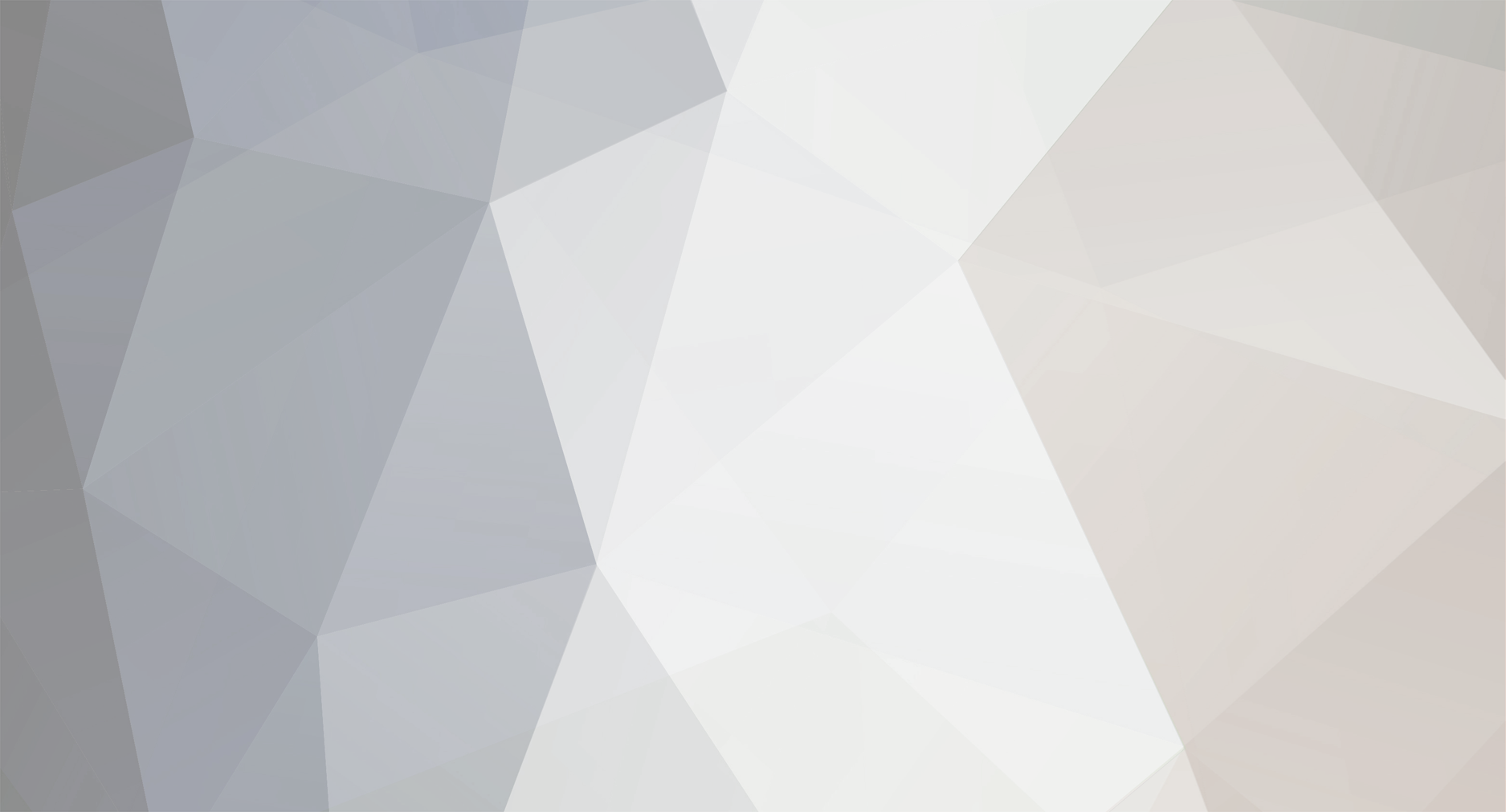
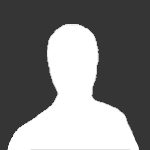
Sherer
Inactive Member-
Posts
112 -
Joined
-
Last visited
-
Days Won
3 -
Feedback
0%
Content Type
Forums
Store
Third Party - Providers Directory
Feature Plan
Release Notes
Docs
Events
Everything posted by Sherer
-
[uitooltip]item value Display cannot be centered!
Sherer replied to Torres's topic in Community Support - Questions & Answers
AlignHorizonalCenter centers all associated objects (from self.childrenList). In case of centering long size TextLines it's better to use AutoAppendTextLine (which tunes the thinboard to the line width). Consider merging those functions from newer uitooltip (I assume you are currently working on old ui): AlignHorizonalCenter AutoAppendTextLine AppendTextLine -
Hello, There is a little memory leak associated with ITEM_BLEND item you would probably like to sort out. Find: LPITEM CHARACTER::AutoGiveItem(DWORD dwItemVnum, WORD bCount, int iRarePct, bool bMsg) Scroll down until you find this kind of code piece: if (item->GetType() == ITEM_BLEND) { for (int i=0; i < INVENTORY_MAX_NUM; i++) { LPITEM inv_item = GetInventoryItem(i); if (inv_item == NULL) continue; if (inv_item->GetType() == ITEM_BLEND) { if (inv_item->GetVnum() == item->GetVnum()) { if (inv_item->GetSocket(0) == item->GetSocket(0) && inv_item->GetSocket(1) == item->GetSocket(1) && inv_item->GetSocket(2) == item->GetSocket(2) && inv_item->GetCount() < ITEM_MAX_COUNT) { inv_item->SetCount(inv_item->GetCount() + item->GetCount()); return inv_item; } } } } } And add there destroy item's function before return statement. The result should look like this: if (item->GetType() == ITEM_BLEND) { for (int i=0; i < INVENTORY_MAX_NUM; i++) { LPITEM inv_item = GetInventoryItem(i); if (inv_item == NULL) continue; if (inv_item->GetType() == ITEM_BLEND) { if (inv_item->GetVnum() == item->GetVnum()) { if (inv_item->GetSocket(0) == item->GetSocket(0) && inv_item->GetSocket(1) == item->GetSocket(1) && inv_item->GetSocket(2) == item->GetSocket(2) && inv_item->GetCount() < ITEM_MAX_COUNT) { inv_item->SetCount(inv_item->GetCount() + item->GetCount()); // Memory Leak Fix M2_DESTROY_ITEM(item); return inv_item; } } } } } Item created above using CreateItem function would never be destroyed at all (it's usually returned from function for further use). Thus you need to wipe it out manually. Regards
-
Other kind of implementation, looking less complicated. Might be useful understanding xP3NG3Rx`s code basing on list`s comprehension: def GetEmptyGridSlot(self, size): SIZE_X = 5 SITE_MAX_NUM = 5 RET_SLOT = -1 ## Setting up grid mtrx = {i : False for i in xrange(player.INVENTORY_PAGE_SIZE*SITE_MAX_NUM)} for i in xrange(player.INVENTORY_PAGE_SIZE*SITE_MAX_NUM): if player.GetItemCount(i) != 0 and mtrx[i] == False: ## Checking grid`s item size if exists item.SelectItem(player.GetItemIndex(i)) (trash, size_y) = item.GetItemSize() ## 'Disabling' grid slot if there is an item for x in xrange(size_y): mtrx[i+(SIZE_X*x)] = True ## Looking for empty slot considering item`s size for i in xrange(player.INVENTORY_PAGE_SIZE*SITE_MAX_NUM): RET_SLOT = i for a in xrange(size): if mtrx[i+(SIZE_X*a)] != False: RET_SLOT = -1 break if RET_SLOT != -1: break return RET_SLOT
-
Indeed. It is just singleton initial call.
-
Added
-
M2 Download Center Download Here ( Internal ) Hello, Working on some new stuff I found out that current implementation of event looks a bit tricky. Due to this fact I basically deciced to re-implement it in C++11 providing up to date tech. Don`t forget to take a look at this topic before you start: [Hidden Content] So lets begin. Add include into the main.cpp: #ifdef __NEW_EVENT_HANDLER__ #include "EventFunctionHandler.h" #endif And add this into main function before: while (idle()); #ifdef __NEW_EVENT_HANDLER__ CEventFunctionHandler EventFunctionHandler; #endif Now add this at the end of idle: #ifdef __NEW_EVENT_HANDLER__ CEventFunctionHandler::instance().Process(); #endif Now search for: sys_log(0, "<shutdown> Destroying CArenaManager..."); And add before: #ifdef __NEW_EVENT_HANDLER__ sys_log(0, "<shutdown> Destroying CEventFunctionHandler..."); CEventFunctionHandler::instance().Destroy(); #endif Now open service.h and add this define: #define __NEW_EVENT_HANDLER__ That`s all. Now just download attachment and add included files to your source.
- 18 replies
-
- 122
-
-
-
-
-
-
#Bump Update added.
-
It is implemented but it is not beeing used
-
That`s a good point but I would rather go into my new solution. If someone (as you say) is beginner this might cost him a lot of troubles.. This release is really out of date so once I find some time I will probably update this topic Using jump triggers is the more practical and newbie-friendly couse` it requires just minimal lua knowledge.
-
That`s linker error. Did you run makedepend before compilation process? If not, you would rather have recompile all object files using this command: gmake clean && gmake Next time when you make any change in the source, dont forget to run makedepend before compilation: gmake dep
-
Hello, I have been working on upgrading some stuff for source compiled on FreeBSD 11.0 32 bits. As you know current libraries are pretty old so I figured out to do some update using the latest sources. Someone may make a use of it. The package contains: - DevIL Library 1.7.8 - JPEG Library 9.1 - Lmcs Library 1.19 - Mng Library 1.0.10 - Png Library 1.6.29 - Tiff Library 1.5 - Mysql C Connector 6.1.9.1 - Jasper Library - Headers for Mysql C Connector and DevIL Due the newer version of DevIL you need to add jasper library to the linker. I did not include Cryptopp Library. If you wanna upgrade it just compile newest version from ports. Link to download: [Hidden Content]
-
Check if you got PercentExp in your CharacterWindow.py file.
-
I cannot get access to this file. Can you upload it on pastebin?
-
Paste your uiCharacter.py here please.
-
game.core Down HELP!!!!!!
Sherer replied to Seryov's topic in Community Support - Questions & Answers
Can you make tops` screen and paste it here? -
game.core Down HELP!!!!!!
Sherer replied to Seryov's topic in Community Support - Questions & Answers
Seems to be just a random crash and you should not care about it. Does it appear often or not? -
game.core Down HELP!!!!!!
Sherer replied to Seryov's topic in Community Support - Questions & Answers
It`s hard to determinate what could be the reason of this crash. Have you got smth else except this core? -
Hello, I have got strange problem with my game file. It crashes like in 15-20 minutes with different reasons which are connected with allocating memory issues. Ram using level is ok, there is a lot of free space, cpu usage is also normal level. Have you got any idea what is going on? Im attaching screen of gdb debbuging: Regards
-
That`s fine. I have seen that a lot of P. Servers are having big issues at the moment. Especially, those which use older game`s revisions.
-
Either your`s and Alpha fixes are ok, yes?
-
M2 Download Center Download Here ( Internal ) Hello! Today, i would like to introduce a new quest trigger - jump. Simple thing, but usefull for people who wants to design more complicated dungeons. Why? F.e: you can add some actions, after warping on eliminated. So, lets start. Open dungeon.cpp and change the struct FWarpToPosition for this one: struct FWarpToPosition { long lMapIndex; long x; long y; FWarpToPosition(long lMapIndex, long x, long y) : lMapIndex(lMapIndex), x(x), y(y) {} void operator()(LPENTITY ent) { if (!ent->IsType(ENTITY_CHARACTER)) { return; } LPCHARACTER ch = (LPCHARACTER)ent; if (!ch->IsPC()) { return; } ch->SaveExitLocation(); if (ch->GetMapIndex() == lMapIndex) { ch->Show(lMapIndex, x, y, 0); ch->Stop(); quest::CQuestManager::instance().Jump(ch->GetPlayerID()); } else { ch->WarpSet(x,y,lMapIndex); } } }; Change also this if statement: if (pDungeon) { pDungeon->JumpAll(m_lMapIndex, m_lWarpX, m_lWarpY); if (!m_stRegenFile.empty()) { pDungeon->SpawnRegen(m_stRegenFile.c_str()); m_stRegenFile.clear(); } } For that: if (pDungeon) { if (!m_stRegenFile.empty()) { pDungeon->SpawnRegen(m_stRegenFile.c_str()); m_stRegenFile.clear(); } pDungeon->JumpAll(m_lMapIndex, m_lWarpX, m_lWarpY); } And its all in dungeon.cpp. Now open quest.h and add: QUEST_JUMP_EVENT, After this: QUEST_ITEM_INFORMER_EVENT, Ok, now you can close file above, and open questmanager.cpp. There add this: m_mapEventName.insert(TEventNameMap::value_type("jump", QUEST_JUMP_EVENT)); Below: m_mapEventName.insert(TEventNameMap::value_type("item_informer", QUEST_ITEM_INFORMER_EVENT)); And simply add also this function anywhere: void CQuestManager::Jump(unsigned int pc) { PC * pPC; if ((pPC = GetPC(pc))) { if (!CheckQuestLoaded(pPC)) return; m_mapNPC[QUEST_NO_NPC].OnJump(*pPC); } else sys_err("QUEST no such pc id : %d", pc); } Thats it. Now we are finished on this file. Open questmanager.h and add function declaration: void Jump(unsigned int pc); After editing questmanager.h, open questnpc.cpp and add this function: bool NPC::OnJump(PC& pc) { return HandleReceiveAllEvent(pc, QUEST_JUMP_EVENT); } And declaration to questnpc.h: bool OnJump(PC& pc); ## UPDATE ## Add this to dungeon.cpp: void CDungeon::JumpAll_NEW(long index, long x, long y) { m_lWarpMapIndex = index; m_lWarpX = x; m_lWarpY = y; event_cancel(&jump_to_event_); dungeon_id_info* info = AllocEventInfo<dungeon_id_info>(); info->dungeon_id = m_id; jump_to_event_ = event_create(dungeon_jump_to_event, info, PASSES_PER_SEC(3)); } And declaration to header: void JumpAll_NEW(long index, long x, long y); And change final call of dungeon_jump_all to this one: pDungeon->JumpAll_NEW(pDungeon->GetMapIndex(), (int)lua_tonumber(L, 1), (int)lua_tonumber(L, 2)); So.. Thats the point. Now look at little example below: when 101.kill with pc.in_dungeon() begin d.jump_all(101, 101) end when jump begin say("Yep, I killed dog and we jumped") end Hope it will help you.
-
[HowTo] Offline Shop System ?
Sherer replied to xLouche's topic in Community Support - Questions & Answers
I doubt that somebody will help you. If you are not able to do this, you can either learn c++ and try yourself or buy it (trade board). -
Shop NPC BUG (source?)
Sherer replied to iRETEMAG's topic in Community Support - Questions & Answers
Some grid? Write my pm, we will fix this problem. -
[Visual BUG]Metin2
Sherer replied to florinrevine's topic in Community Support - Questions & Answers
Add in char_affect.cpp, in RemoveAffect function UpdatePacket in else AFFECT_REVIVE_INVISIBLE instruction.- 1 reply
-
- 1
-
-
Shop NPC BUG (source?)
Sherer replied to iRETEMAG's topic in Community Support - Questions & Answers
Did you change something in py files of shops? Or in source?