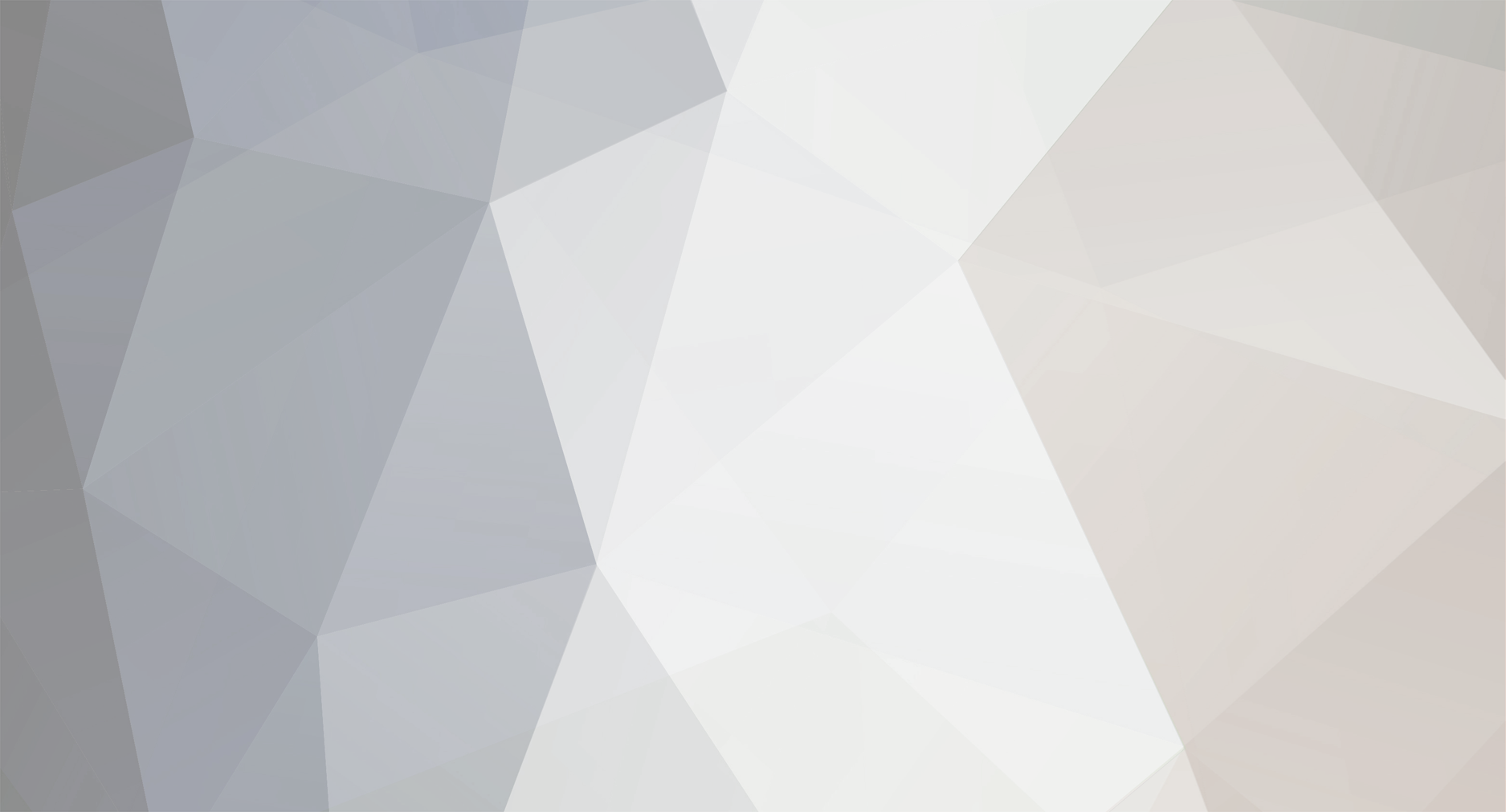
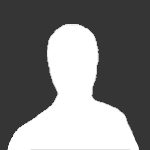
foreach
-
Posts
2 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Forums
Store
Third Party - Providers Directory
Feature Plan
Release Notes
Docs
Events
Posts posted by foreach
-
-
Version of Files : XXX
Hello
lately we've been stuck on compiling source. The details are in this post:
We are clueless and we will give 20 eur to who will show us how to successfully compile source.
Thank you in advance,
foreach
[reward] source compiling
in Community Support - Questions & Answers
Posted
Hello, sorry for late answer.
This is what i found, but im not very confident in my knowledne in this area. My friend can tell you more after he wakes up