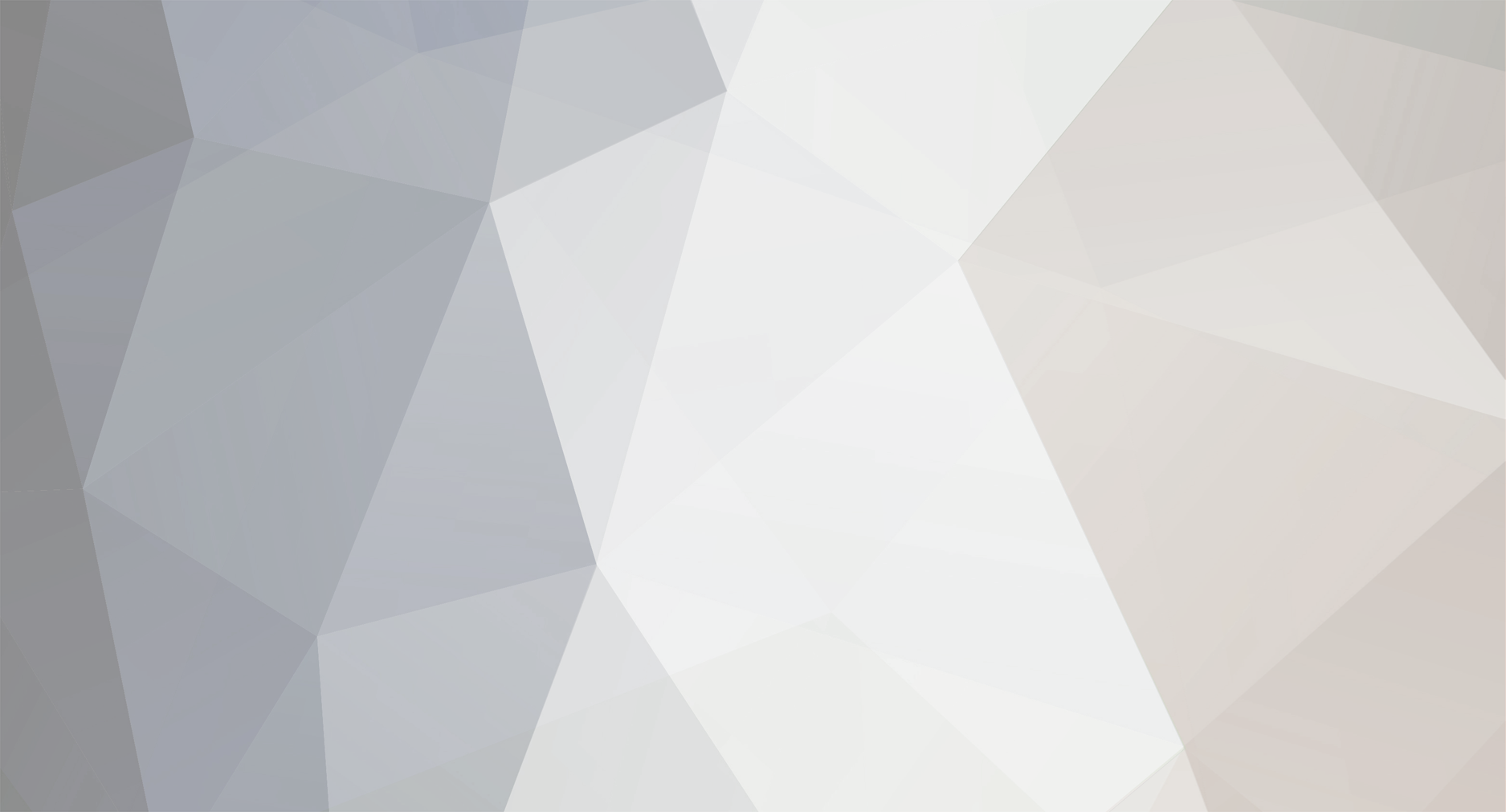
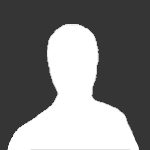
Foxxielove
-
Posts
24 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Forums
Store
Third Party - Providers Directory
Feature Plan
Release Notes
Docs
Events
Posts posted by Foxxielove
-
-
12 hours ago, HFWhite said:
Do you have a video/gif of the system ? I don;t really understand what it does.
When you are within a certain radius of NPC x, your Auto Potion will be refilled. This means if your Auto Potion only has 5% remaining, it will regenerate/refill your potion.
-
-
I have also the problem with the connection.
When I try to connect via Client the server is offline but started without problems.
btw: my ports are fine and not blocked/closed
-
thanks I'll try it.
-
anyone can help me with the introloading?
I have the renewal from vegas but some functions are not like the old one.
my file
Spoiler#! /usr/bin/env python __author__ = 'VegaS' __date__ = '2020-02-04' __name__ = 'IntroLoading Renewal' __version__ = '4.3' import ui import uiScriptLocale import net import app import player import background import wndMgr import constInfo import playerSettingModule import colorInfo import chrmgr import localeInfo import emotion import playerLoad from _weakref import proxy ################################## ## loadingFunctions ################################## NAME_COLOR_DICT = { chrmgr.NAMECOLOR_PC : colorInfo.CHR_NAME_RGB_PC, chrmgr.NAMECOLOR_NPC : colorInfo.CHR_NAME_RGB_NPC, chrmgr.NAMECOLOR_MOB : colorInfo.CHR_NAME_RGB_MOB, chrmgr.NAMECOLOR_PVP : colorInfo.CHR_NAME_RGB_PVP, chrmgr.NAMECOLOR_PK : colorInfo.CHR_NAME_RGB_PK, chrmgr.NAMECOLOR_PARTY : colorInfo.CHR_NAME_RGB_PARTY, chrmgr.NAMECOLOR_WARP : colorInfo.CHR_NAME_RGB_WARP, chrmgr.NAMECOLOR_WAYPOINT : colorInfo.CHR_NAME_RGB_WAYPOINT, chrmgr.NAMECOLOR_BOSS : colorInfo.CHR_NAME_RGB_BOSS, chrmgr.NAMECOLOR_EMPIRE_MOB : colorInfo.CHR_NAME_RGB_EMPIRE_MOB, chrmgr.NAMECOLOR_EMPIRE_NPC : colorInfo.CHR_NAME_RGB_EMPIRE_NPC, chrmgr.NAMECOLOR_EMPIRE_PC+1 : colorInfo.CHR_NAME_RGB_EMPIRE_PC_A, chrmgr.NAMECOLOR_EMPIRE_PC+2 : colorInfo.CHR_NAME_RGB_EMPIRE_PC_B, chrmgr.NAMECOLOR_EMPIRE_PC+3 : colorInfo.CHR_NAME_RGB_EMPIRE_PC_C, } if app.ENABLE_OFFLINESHOP_SYSTEM: NAME_COLOR_DICT[chrmgr.NAMECOLOR_SHOP] = colorInfo.CHR_NAME_RGB_SHOP TITLE_COLOR_DICT = ( colorInfo.TITLE_RGB_GOOD_4, colorInfo.TITLE_RGB_GOOD_3, colorInfo.TITLE_RGB_GOOD_2, colorInfo.TITLE_RGB_GOOD_1, colorInfo.TITLE_RGB_NORMAL, colorInfo.TITLE_RGB_EVIL_1, colorInfo.TITLE_RGB_EVIL_2, colorInfo.TITLE_RGB_EVIL_3, colorInfo.TITLE_RGB_EVIL_4, ) if app.ENABLE_STONEMINIMAP: NAME_COLOR_DICT.update({ chrmgr.NAMECOLOR_METIN : colorInfo.CHR_NAME_RGB_METIN, }) def __main__(): ## RegisterColor if app.TOURNAMENT_PVP_SYSTEM: NAME_COLOR_DICT.update({chrmgr.NAMECOLOR_TOURNAMENT_TEAM_A : (255, 31, 31),}) NAME_COLOR_DICT.update({chrmgr.NAMECOLOR_TOURNAMENT_TEAM_B : (10, 135, 245),}) for nameIndex, nameColor in NAME_COLOR_DICT.items(): chrmgr.RegisterNameColor(nameIndex, *nameColor) for titleIndex, titleColor in enumerate(TITLE_COLOR_DICT): chrmgr.RegisterTitleColor(titleIndex, *titleColor) ## RegisterTitleName for titleIndex, titleName in enumerate(localeInfo.TITLE_NAME_LIST): chrmgr.RegisterTitleName(titleIndex, titleName) ## RegisterEmotionIcon emotion.RegisterEmotionIcons() ## RegisterDungeonMapName dungeonMapNameList = ("metin2_map_spiderdungeon", "metin2_map_monkeydungeon", "metin2_map_monkeydungeon_02", "metin2_map_monkeydungeon_03", "metin2_map_deviltower1") for dungeonMapName in dungeonMapNameList: background.RegisterDungeonMapName(dungeonMapName) ## LoadGuildBuilding playerSettingModule.LoadGuildBuildingList(localeInfo.GUILD_BUILDING_LIST_TXT) if app.RACE_HEIGHT: playerSettingModule.LoadRaceHeight() ################################## ## LoadingWindow ################################## class LoadingWindowNew(ui.AniImageBox): def __del__(self): ui.AniImageBox.__del__(self) net.SetPhaseWindow(net.PHASE_WINDOW_LOAD, 0) def __init__(self, stream): ui.AniImageBox.__init__(self) net.SetPhaseWindow(net.PHASE_WINDOW_LOAD, self) self.Destroy() self.stream = proxy(stream) for j in xrange(14): self.AppendImage("d:/ymir work/ui/loading_img/%d.tga"%j) self.SetCenterPosition() def Destroy(self): self.stream = None def Open(self): self.Show() net.SendSelectCharacterPacket(self.stream.GetCharacterSlot()) def Close(self): self.Hide() def DEBUG_LoadData(self, playerX, playerY): self.LoadData(playerX, playerY) def LoadData(self, playerX, playerY): playerLoad.RegisterSkill(net.GetMainActorRace(), net.GetMainActorSkillGroup(), net.GetMainActorEmpire()) background.SetViewDistanceSet(background.DISTANCE0, 25600) background.SelectViewDistanceNum(background.DISTANCE0) app.SetGlobalCenterPosition(playerX, playerY) net.StartGame() class LoadingWindow(ui.ScriptWindow): def __init__(self, stream): ui.Window.__init__(self) net.SetPhaseWindow(net.PHASE_WINDOW_LOAD, self) self.stream = proxy(stream) def __del__(self): net.SetPhaseWindow(net.PHASE_WINDOW_LOAD, 0) ui.Window.__del__(self) def Open(self): net.SendSelectCharacterPacket(self.stream.GetCharacterSlot()) app.SetFrameSkip(0) self.Show() def Close(self): app.SetFrameSkip(1) self.Hide() def OnPressEscapeKey(self): app.SetFrameSkip(1) self.stream.SetLoginPhase() return True def DEBUG_LoadData(self, playerX, playerY): self.LoadData(playerX, playerY) def LoadData(self, playerX, playerY): playerLoad.RegisterSkill(net.GetMainActorRace(), net.GetMainActorSkillGroup(), net.GetMainActorEmpire()) background.SetViewDistanceSet(background.DISTANCE0, 25600) background.SelectViewDistanceNum(background.DISTANCE0) app.SetGlobalCenterPosition(playerX, playerY) net.StartGame()
vegas title system file
Spoiler# 1) Search: count = 0 for rgb in TITLE_COLOR_DICT: chrmgr.RegisterTitleColor(count, rgb[0], rgb[1], rgb[2]) count += 1 # 2) After their function make a new line and paste: # NEW TITLE SYSTEM - VEGAS if app.ENABLE_TITLE_SYSTEM: TITLEPRESTIGE_COLOR_DICT = ( colorInfo.TITLE_PRESTIGE_COLOR_1, colorInfo.TITLE_PRESTIGE_COLOR_2, colorInfo.TITLE_PRESTIGE_COLOR_3, colorInfo.TITLE_PRESTIGE_COLOR_4, colorInfo.TITLE_PRESTIGE_COLOR_5, colorInfo.TITLE_PRESTIGE_COLOR_6, colorInfo.TITLE_PRESTIGE_COLOR_7, colorInfo.TITLE_PRESTIGE_COLOR_8, colorInfo.TITLE_PRESTIGE_COLOR_9, colorInfo.TITLE_PRESTIGE_COLOR_10, colorInfo.TITLE_PRESTIGE_COLOR_11, colorInfo.TITLE_PRESTIGE_COLOR_12, colorInfo.TITLE_PRESTIGE_COLOR_13, colorInfo.TITLE_PRESTIGE_COLOR_14, colorInfo.TITLE_PRESTIGE_COLOR_15, colorInfo.TITLE_PRESTIGE_COLOR_16, colorInfo.TITLE_PRESTIGE_COLOR_17, colorInfo.TITLE_PRESTIGE_COLOR_18, colorInfo.TITLE_PRESTIGE_COLOR_19, colorInfo.TITLE_PRESTIGE_COLOR_0,) count_prestige_vegas = 0 for rgb in TITLEPRESTIGE_COLOR_DICT: chrmgr.RegisterTitlePrestigeColor(count_prestige_vegas, rgb[0], rgb[1], rgb[2]) count_prestige_vegas += 1 # 1) Search: self.__RegisterTitleName() # 2) After their function make a new line and paste: if app.ENABLE_TITLE_SYSTEM: self.__RegisterTitlePrestigeName() """""""""""""""""""""""""""""""""""""""""" # 1) Search: def __RegisterTitleName(self): # 2) After their function make a new line and paste: if app.ENABLE_TITLE_SYSTEM: def __RegisterTitlePrestigeName(self): for i in xrange(len(localeInfo.TITLEPRESTIGE_NAME_LIST)): chrmgr.RegisterTitlePrestigeName(i, localeInfo.TITLEPRESTIGE_NAME_LIST[i]) """"""""""""""""""""""""""""""""""""""""""
-
really nice.
how we can improve it only with P skill or an item? is there a easy way? -
Hey, I used your vdi for testing and the first try it work all fine.
1 day later I started the server and got connection refused
In every core, auth
DB syserr is fine
SYSERR: Nov 5 23:20:13 :: socket_connect: HOST 127.0.0.1:15000, could not connect. DB: SYSERR: Nov 5 23:20:38 :: Start: TABLE_POSTFIX not configured use default SYSERR: Nov 5 23:22:54 :: pid_init: Start of pid: 918 SYSERR: Nov 5 23:22:54 :: Start: TABLE_POSTFIX not configured use default SYSERR: Nov 5 23:23:43 :: hupsig: SIGHUP, SIGINT, SIGTERM signal has been received. shutting down. SYSERR: Nov 5 23:23:43 :: pid_deinit: End of pid
when I start all manually it works sometimes but 90% I got connection refused.
-
8 hours ago, NonnaPelata2 said:
I'm having trouble downloading the "client - serverfiles" and "VDI", after a few minutes of downloading i get the err: "Operation failed. Network error" with the other files this not happend
try with another browser.
I think u use chrome, correct?
-
syserr?
maybe u repacked your textureset wrong or with an other key?
-
On 11/12/2022 at 3:53 AM, БlyatMAN said:
i fixed with this set GLOBAL sql_mode='NO_ENGINE_SUBSTITUTION' but after i reboot sv i must do this again
write this in your my.cnf
-
On 11/3/2022 at 3:25 PM, lordzokarum said:
Found an interesting situation.
1. Open Character Status window (click c)
2. click on Defence increase button (the + plus button) = Defence is NOT increasing
3. click on HP increase button (the + plus button) = HP increases BUT Defence increases TOOcan anyone help??
Thanks
VIT/HP increase def but where u can status up your def? :D
Vit, Int, Str, Dex idk why u talk about def status up
-
any one know what I did wrong?
It doesn't load the icons but the tga files are located in icon/emoji/
-
1
-
-
game.py is in the root pack file.
just unpack your root files and then u can edit them.
-
On 10/22/2022 at 6:29 PM, ReFresh said:
Did you checked coordinates, if they're not colliding with another map? I recommend you to use Atlas Manager. And what about server part?
Yeah I did.
The coordinates doesn't colidate with another map.
Serverpart is the same as client - i just deleted the area folders and just uploaded the setting.txt and server_attr and created new txt files like town.txt
-
3 hours ago, ReFresh said:
I had this error many times and I always fixed it. It can be, because you wrongly added map to atlasinfo with wrong coordinates, it can be nonexisting path to the map folder, wrongly packed files like property one, because of folders, which contain ÆÄÀÏÀÌ ¾øÀ» °¡´É¼ºÀÌ ¸¹½À´Ï´Ù characters, which not every packer can pack correctly. Etc. it's really common error, which you should be able to fix.
thanks for ur answer
my property is fine without special characters I checked them and its every new map.. I tried 5 maps and all of them doesn't work.
my atlasinfo
metin2_patch_dungeons/metin2_map_picabo 1547900 1222900 2 2 metin2_plechi/plant_dungeon 133900 133900 3 3
my pack files
Index File metin2_map_picabo/ metin2_patch_dungeons
-
Hey there,
I have a problem with a new map... an other User here had the same problem many years ago.
1020 17:43:13142 :: CMapBase::LoadProperty(FileName=metin2_patch_dungeons/picabo\MapProperty.txt) - LoadMultipleTextData ERROR ÆÄÀÏÀÌ ¾øÀ» °¡´É¼ºÀÌ ¸¹½À´Ï´Ù. 1020 17:43:13142 :: CMapManager::LoadMap() Invalid Map Type 1020 17:43:13142 :: CPythonBackground::SelectViewDistanceNum(int eNum=0) mc_pcurEnvironmentData is NULL
I created a new pack file names metin2_patch_dungeons with the data files from the map.
After the error I put the zone, property and and and files in the old original pack files and only the map datas in a new pack file but doesn't work too.
Any one have a fix or an idea?
kind regards
-
I tried the 4th inventory pages and it works serversided without problems but ingame its a bit strange..
equip is empty and the potts from my belt inventory shows now on the last page.
idk what I did wrong in the python scripts
-
it works without problems, thanks
-
-
-
hey there i just wanna save a server attr for my 64x64 map.
when i wanna save it the WE gives me a message "out of memory"
is there a way to fix it?
Shop Ex Renewal
in Features & Metin2 Systems
Posted · Edited by Metin2 Dev International
Core X - External 2 Internal
It works fine but have an issue with cheque system.
I can buy the item for 250 yang but it shows won too.
Anyone have a solution?