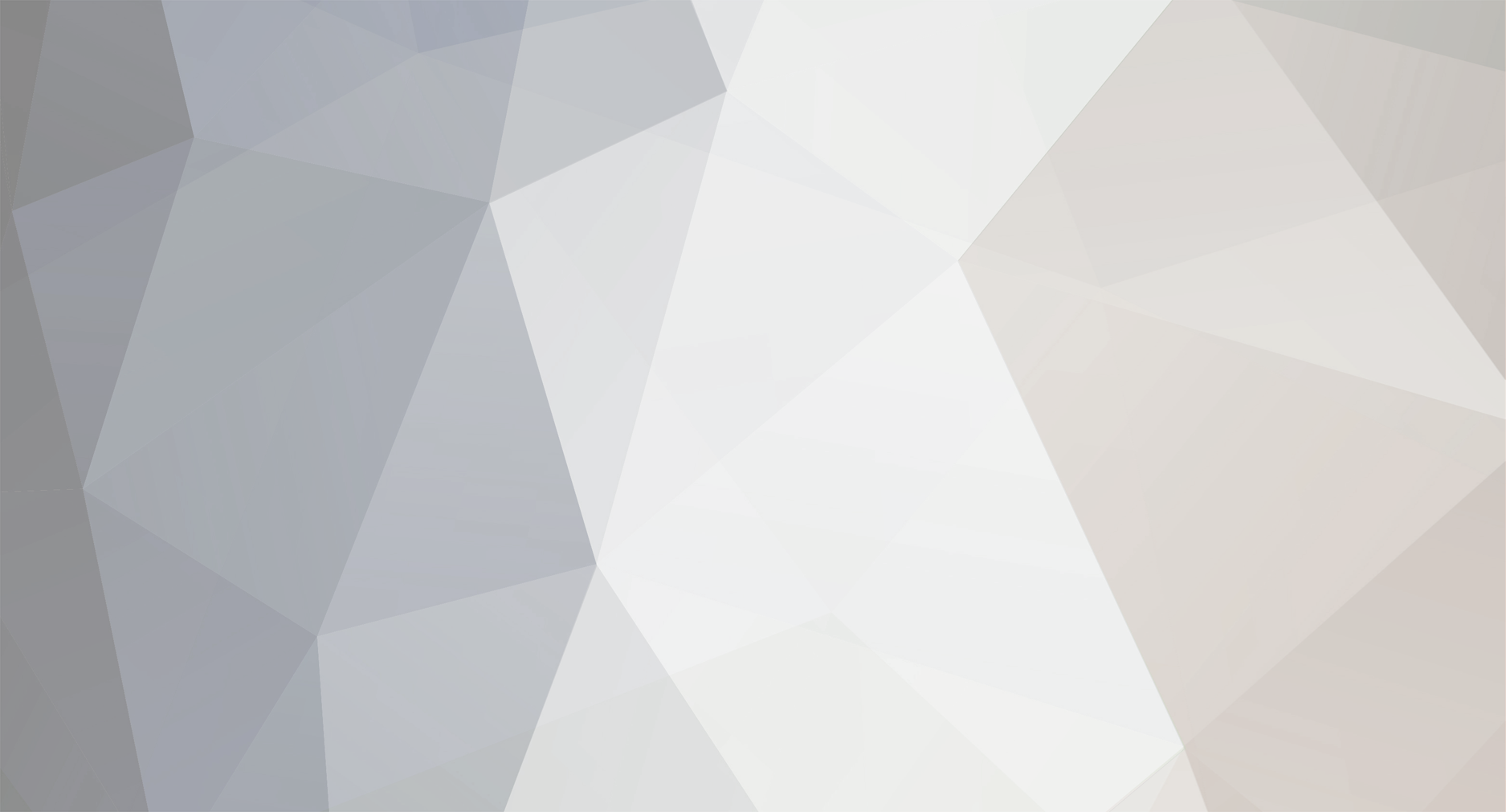
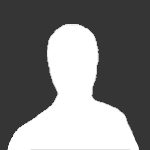
Seryov
-
Posts
89 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Forums
Store
Third Party - Providers Directory
Feature Plan
Release Notes
Docs
Events
Posts posted by Seryov
-
-
Can you share libs or tuturial for compile in Windows Server?
-
1
-
-
uint16_t CHARACTER::FindCharacterVID(const char* name) { const LPCHARACTER ch = CHARACTER_MANAGER::Instance().FindPC(name); return ch ? ch->GetVID() : 0; }
not tested.
-
Hi, i m searching this effect, someone know what is?
-
Please, can someone decrypt and upload full GF lasted client?
-
Could someone help me, where is the fixed bonuses of weapons located in the source?
I found the armor located at char.cpp : ComputeBattlePoints(), , but I can't find where the weapon's fixed bonuses are calculated.
Thank you all. -
17 hours ago, xP3NG3Rx said:
As far as I know this class controlled by the OX event timers. The base of it is on the server and the processor is inside the binary.
ps.: of course it can be handled from python too.
Thank you so much xP3NG3Rx!!! -
Hello, I'm testing the class "BigBoardControl"
Code:
class BigBoardControl(ui.Bar): FONT_WIDTH = 18 FONT_HEIGHT = 18 LINE_WIDTH = 500 LINE_HEIGHT = FONT_HEIGHT + 5 STEP_HEIGHT = LINE_HEIGHT * 2 LINE_CHANGE_LIMIT_WIDTH = 450 BIG_TEXTBAR_MAX_HIGHT = 1000 FRAME_IMAGE_FILE_NAME_LIST = [ "season1/interface/oxevent/frame_new_0.sub", "season1/interface/oxevent/frame_new_1.sub", ] FRAME_IMAGE_STEP = 256 FRAME_BASE_X = -20 FRAME_BASE_Y = -12 def __init__(self): ui.Bar.__init__(self) self.AddFlag("not_pick") self.tipList = [] self.curPos = 0 self.dstPos = 0 self.nextScrollTime = 0 self.scrollstop = 0 self.pretexsize = 0 self.nexttextsize = 0 self.changeline = 0 self.addtipListcount = 0 self.SetPosition(0, 150) self.SetSize(512, 55) self.SetColor(grp.GenerateColor(0.0, 0.0, 0.0, 0.5)) self.SetWindowHorizontalAlignCenter() self.__CreateTextBar() self.__LoadFrameImages() def __LoadFrameImages(self): x = self.FRAME_BASE_X y = self.FRAME_BASE_Y self.imgList = [] for imgFileName in self.FRAME_IMAGE_FILE_NAME_LIST: self.imgList.append(self.__LoadImage(x, y, imgFileName)) def __LoadImage(self, x, y, fileName): img = ui.ImageBox() img.SetParent(self) img.AddFlag("not_pick") img.LoadImage(fileName) img.SetPosition(x, y) img.Show() return img def __del__(self): self.tipList = [] self.textBar.ClearBar() self.Hide() ui.Bar.__del__(self) def __CreateTextBar(self): x, y = self.GetGlobalPosition() self.textBar = BigTextBar(self.LINE_WIDTH, self.BIG_TEXTBAR_MAX_HIGHT, self.FONT_HEIGHT) self.textBar.SetParent(self) self.textBar.SetPosition(6, 8) self.textBar.SetTextColor(242, 231, 193) self.textBar.SetClipRect(0, y+8, wndMgr.GetScreenWidth(), y+8+self.STEP_HEIGHT) self.textBar.Show() def __CleanOldTip(self): self.tipList = [] self.textBar.ClearBar() self.Hide() def __RefreshBoard(self): self.textBar.ClearBar() preaddtipListcount = self.addtipListcount self.addtipListcount = 0 index = 0 for text in self.tipList: (text_width, text_height) = self.textBar.GetTextExtent(text) if index == 0: self.textBar.TextOut((500-text_width)/2, index*self.LINE_HEIGHT, " ") index += 1 self.addtipListcount += 1 if index == (len(self.tipList) - (len(self.tipList) - self.pretexsize))+1 and not self.pretexsize == 0: self.textBar.TextOut((500-text_width)/2, index*self.LINE_HEIGHT, " ") index += 1 self.textBar.TextOut((500-text_width)/2, index*self.LINE_HEIGHT, " ") index += 1 self.addtipListcount += 2 self.textBar.TextOut((500-text_width)/2, index*self.LINE_HEIGHT, text) index += 1 if self.tipList: index += 1 self.addtipListcount += 1 self.textBar.TextOut((500-text_width)/2, index*self.LINE_HEIGHT, " ") def SetTip(self, text): if not app.IsVisibleNotice(): return if text == "#start" or text == "#end" or text == "#send": pass else: self.__AppendText(text) if text == "#start" or text == "#send": if text == "#send" and self.pretexsize == 0: text = "#end" self.__RefreshBoard() if text == "#start": self.STEP_HEIGHT = (self.LINE_HEIGHT * (len(self.tipList) + self.addtipListcount)) self.pretexsize = len(self.tipList) x, y = self.GetGlobalPosition() self.textBar.SetClipRect(0, y+8, wndMgr.GetScreenWidth(), y+8+self.STEP_HEIGHT) self.SetSize(512, 10 + (self.LINE_HEIGHT * (len(self.tipList) + self.addtipListcount))+10) self.imgList[1].SetPosition(self.FRAME_BASE_X, self.STEP_HEIGHT + 10) self.scrollstop = 0 self.changeline = 1 if text == "#send": self.scrollstop = 1 self.nexttextsize = len(self.tipList) - self.pretexsize if not self.IsShow(): self.curPos = -self.STEP_HEIGHT self.dstPos = -self.STEP_HEIGHT self.textBar.SetPosition(3, 8 - self.curPos) self.Show() if text == "#end": self.curPos = -self.STEP_HEIGHT self.dstPos = -self.STEP_HEIGHT self.textBar.SetPosition(3, 8 - self.curPos) self.textcount = 0 self.changeline = 0 self.pretexsize = 0 self.nexttextsize = 0 self.addtipListcount = 0 self.__CleanOldTip()
I made:def Test(self): self.BINARY_SetBigControlMessage("message1") self.BINARY_SetBigControlMessage("message1") self.BINARY_SetBigControlMessage("#send")
However, the code does not pause, it goes straight as soon as it shows the message, does anyone have any idea how this code works correctly?
Originally, it shows the message, stops at the message and then continues.
Thanks.
Quote@EDITED:
Works, I just removed the line:
self.BINARY_SetBigControlMessage("#send") -
awesome topic, congratulations.
-
I have this problem, and I want to pay someone to fix it for me.
It's like a ghost drop item, I dont have Idea. -
Thanks man!
-
Does anyone know what happened to WoM2 and WoM3?
-
Someone know how to fix this problem for AMD VISHERA users?
Thanks.
-
I had the same problem with the paid version of the great offline shop.
-
Please i'm searching c++ coder for easy works, send me pm.
-
On 8/30/2019 at 11:11 AM, WeedHex said:
locale/x/ui
Yes, but if you set 0, the equipped items don't appear, I think is needed to change client source part, someone know how?
-
Someone know how to change client to start EQUIPMENT_START_INDEX on index 0 without 180 for 4/inventory?
Thank you all. -
Try this:
background.SetTransparentTree(0);
must be 0 or 1 -
This problem is due to guild objects, note that it will only happen in the main villages.
If you disable sequence, the error will be numbered to 100.
This error happens because guild objects are loaded before maps are loaded, they are also loaded in core that do not have maps allowed, causing others errors.
Note that if you clear all guild objects the problem will stop.
I don't know a solution for this if you find any share it with us.
-
Hello,
I'm trying to call the tabs of new pet window.
window["children"] = window["children"] + [ { "name" : "PetAttrChange_Page", "type" : "window", "style" : ("attach",), "x" : 0, "y" : 0, "width" : 352, "height" : 408, "children" : [ ## Pet Attr Change UI BG { "name" : "PetAttrChangeUIBG", "type" : "expanded_image", "style" : ("attach",), "x" : 0, "y" : 0, "image" : "d:/ymir work/ui/pet/res/Pet_UI_bg2.tga" }, ## Pet Information Title { "name" : "TitleAttrChangeWindow", "type" : "window", "x" : 10, "y" : 10, "width" : PET_UI_BG_WIDTH-10-15, "height" : 15, "style" : ("attach",), "children" : ( {"name":"TitleName", "type":"text", "x":0, "y":0, "text":uiScriptLocale.PET_INFORMATION_TITLE, "all_align" : "center"}, ), }, ## Close Button { "name" : "ClosePetChangeWndButton", "type" : "button", "x" : PET_UI_BG_WIDTH -10-15, "y" : 10, "tooltip_text" : uiScriptLocale.CLOSE, "default_image" : "d:/ymir work/ui/public/close_button_01.sub", "over_image" : "d:/ymir work/ui/public/close_button_02.sub", "down_image" : "d:/ymir work/ui/public/close_button_03.sub", }, (...)
I did like this:
wndPetInfoPage = self.GetChild("PetInfo_Page")
wndPetAttrChange = self.GetChild("PetAttrChange_Page")
I have this syserr:
petInformationWindow.LoadWindow.BindObject - <type 'exceptions.KeyError'>:'PetAttrChange_Page'
Someone know why? -
Could someone post any current open client with the name of every patch just like the P3NG3R does?
@xP3NG3Rx -
Hello, I have a problem with my server probably in the Cache, my game core crashed in random ways in the "SaveSingleItem" function, so I made this correction:
Spoilervoid ITEM_MANAGER::SaveSingleItem(LPITEM item) { if (!item->GetOwner()) { DWORD dwID = item->GetID(); DWORD dwOwnerID = item->GetLastOwnerPID(); db_clientdesc->DBPacketHeader(HEADER_GD_ITEM_DESTROY, 0, sizeof(DWORD) + sizeof(DWORD)); db_clientdesc->Packet(&dwID, sizeof(DWORD)); db_clientdesc->Packet(&dwOwnerID, sizeof(DWORD)); sys_log(1, "ITEM_DELETE %s:%u", item->GetName(), dwID); return; } if (!item) return; if (!item->GetOwner()) return; if (item->GetCount() < 1) return; if (item->GetCount() > 200) return; if (item->GetOriginalVnum() < 1) return; if (item->GetOriginalVnum() > 9999999) return; .....
Because the SaveSingleItem function returned null values, such as owner, vnum, and other things.....
But the problem doenst solved, now my db is crashing with another problem...
GDB:Spoiler
I paid for someone able to help me fix these problems.
-
Help, I pay for the fix.
-
Someone know how to read this value in quest or c++?
ADDON_TYPE0 ADDON_VALUE0<<<
Thanks -
8 hours ago, flexio said:
sysser,syslog after crash?
which version offline shops you have?
Great Offline Shop
No syserr or syslog
Proper SIGNAL management for Windows
in Bug Fixes
Posted
I usually close the cores using ESC in Windows, with this change is it now possible to close using CTRL + C?