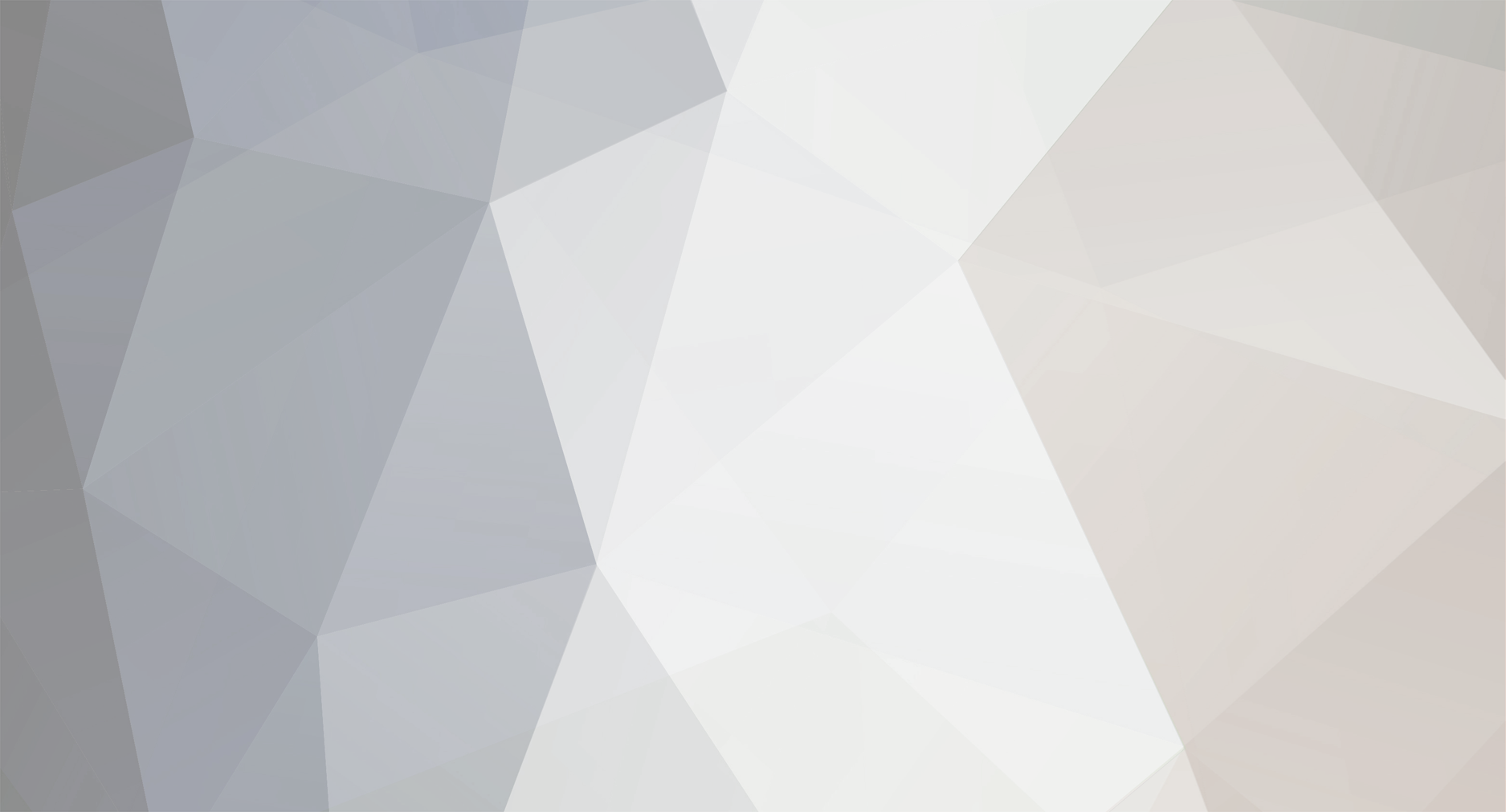
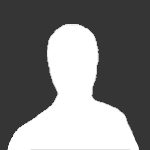
Jira
-
Posts
191 -
Joined
-
Days Won
2 -
Feedback
0%
Content Type
Forums
Store
Third Party - Providers Directory
Feature Plan
Release Notes
Docs
Events
Posts posted by Jira
-
-
item drop penalty
source/server/game/char_battle.cpp | 12 +++++++++++- 1 file changed, 11 insertions(+), 1 deletion(-) diff --git a/source/server/game/char_battle.cpp b/source/server/game/char_battle.cpp index 4b0c013..9f9fab6 100644 --- a/source/server/game/char_battle.cpp +++ b/source/server/game/char_battle.cpp @@ -1107,8 +1107,18 @@ void CHARACTER::ItemDropPenalty(LPCHARACTER pkKiller) std::vector<BYTE> vec_bSlots; for (i = 0; i < INVENTORY_MAX_NUM; ++i) - if (GetInventoryItem(i)) + { + if ((pkItem = GetInventoryItem(i))) + { + if (pkItem->GetType() == ITEM_QUEST) + { + if (quest::CQuestManager::instance().GetPCForce(GetPlayerID())->IsRunning() == true) + continue; + } + vec_bSlots.push_back(i); + } + } if (!vec_bSlots.empty()) {
-
1
-
-
Thanks.
Observer mode has the same bug.
-
If you send the move and attack packet to target and if you have the same position as the target then this check is pointless.
An extra check that you can add
if (fHittingDistance == 0.0f && victim->GetX() == ch->GetX() && victim->GetY() == ch->GetY() && !victim->IsPC()) return;
-
1
-
-
It also has a memory leak
The name of the timer is never deallocated when CancelServerTimers or ClearServerTimer is called
Spoilerdiff --git a/game/src/questevent.cpp b/game/src/questevent.cpp index 21fa0ed5b5a3fa3d0572494137602c2ee5cbc2c4..2a6cf3f73c7732d4d67c0bf044d510f1225ceaa8 100644 --- a/game/src/questevent.cpp +++ b/game/src/questevent.cpp @@ -20,6 +20,19 @@ namespace quest event_cancel(ppEvent); } + void CancelServerTimerEvent(LPEVENT* ppEvent) + { + quest_server_event_info* info = dynamic_cast<quest_server_event_info*>((*ppEvent)->info); + + if (info) + { + delete [] (info->name); + info->name = NULL; + } + + event_cancel(ppEvent); + } + EVENTFUNC(quest_server_timer_event) { quest_server_event_info * info = dynamic_cast<quest_server_event_info *>( event->info ); diff --git a/game/src/questevent.h b/game/src/questevent.h index 2be83a0106e2f01cc8d1f50e7f2779957842c176..76500b52fd10d8f22965920f1a9d234b91b7ead3 100644 --- a/game/src/questevent.h +++ b/game/src/questevent.h @@ -37,4 +37,5 @@ namespace quest extern LPEVENT quest_create_server_timer_event(const char* name, double when, unsigned int timernpc = QUEST_NO_NPC, bool loop = false, unsigned int arg = 0); extern LPEVENT quest_create_timer_event(const char* name, unsigned int player_id, double when, unsigned int npc_id=QUEST_NO_NPC, bool loop = false); extern void CancelTimerEvent(LPEVENT* ppEvent); -} \ No newline at end of file + extern void CancelServerTimerEvent(LPEVENT* ppEvent); +} diff --git a/game/src/questmanager.cpp b/game/src/questmanager.cpp index 2e58b776c4c3af97d2d0654d227d239ff177bf32..c2ed5eca1352f79f87003f7382b82db8f2682fd3 100644 --- a/game/src/questmanager.cpp +++ b/game/src/questmanager.cpp @@ -21,6 +21,7 @@ #include "dungeon.h" #include "regen.h" +#include "questevent.h" @@ -1853,7 +1854,7 @@ namespace quest if (it != m_mapServerTimer.end()) { LPEVENT event = it->second; - event_cancel(&event); + CancelServerTimerEvent(&event); m_mapServerTimer.erase(it); } @@ -1864,7 +1865,7 @@ namespace quest auto it = m_mapServerTimer.begin(); while (it != m_mapServerTimer.end()) { if (it->first.second == arg) { - event_cancel(&it->second); + CancelServerTimerEvent(&it->second); it = m_mapServerTimer.erase(it); continue; }
-
1
-
-
Thanks, I'm so curious if can be exploitable with net.SendChangeNamePacket
-
I think you meant to do like this, with your fix you cannot drop gold.
void CInputMain::ItemDrop2(LPCHARACTER ch, const char * data) { TPacketCGItemDrop2 * pinfo = (TPacketCGItemDrop2 *) data; if (!ch) return; if (pinfo->gold > 0) ch->DropGold(pinfo->gold); else { LPITEM pkItem = ch->GetItem(pinfo->Cell); if (!pkItem) return; if (pkItem->IsEquipped()) return; ch->DropItem(pinfo->Cell); } }
But it's better to check inside of CHARACTER::DropItem function. Btw don't use macros it's enough to put a comment like // FIXME: can't drop equipment
-
just add -Wno-invalid-source-encoding
ex)
CFLAGS = -m32 -g -Wall -O2 -pipe -fexceptions -std=c++17 -fno-strict-aliasing -pthread -D_THREAD_SAFE -DNDEBUG -Wno-invalid-source-encoding
-
7 minutes ago, Draveniou1 said:
To answer so means you have no idea of programming
Yes you're a big programmer (idiot). Give me on dm that server with 1k players I'll make that server to cry.
-
1
-
-
20-30 days?
Dmn pls somebody ban this idiot and delete this shit. For you're secure server really I don't recommend to remove te improved encryption (that's why is called 'IMPROVED').
-
43 minutes ago, Draveniou1 said:
Need 1 example
EXAMPLE: CLIENTSIDE: typedef struct command_login3 { BYTE header; char name[ID_MAX_NUM + 1]; char pwd[PASS_MAX_NUM + 1]; DWORD adwClientKey[4]; #ifdef PROTECT_KEY_FROM_HACKERS long 28374235784954363253456345346346345634563456678678977823834681293345634563456675674784784574124792375237; //max hacker 255 :) not unlock #endif } TPacketCGLogin3; SERVERSIDE: typedef struct command_login3 { BYTE header; char login[LOGIN_MAX_LEN + 1]; char passwd[PASSWD_MAX_LEN + 1]; DWORD adwClientKey[4]; #ifdef PROTECT_KEY_FROM_HACKERS long 28374235784954363253456345346346345634563456678678977823834681293345634563456675674784784574124792375237; #endif } TPacketCGLogin3; SERVICE CLIENT/SERVER #define PROTECT_KEY_FROM_HACKERS Hackers can not find more than 250 BYTES as the number 28374235784954363253456345346346345634563456678678977823834681293345634563456675674784784574124792375237 It will protect you
I can find that key in 1min. What are you doing here is a bad practice, you can trick just the kids.
-
1
-
-
On 10/1/2021 at 6:23 PM, zsoltiabeka10 said:
Hey guys, anyone knows how can I get characters like "á,ő,ú,ű" etc working? Right now it's displayed as "?".
I tried changing file encoding but it didn't work for me.
Thanks in advance!https://docs.microsoft.com/en-us/cpp/preprocessor/execution-character-set?view=msvc-160
-
1
-
-
for client crash
void CPythonApplication::__ResetCameraWhenMinimize() { if (m_CursorHandleMap.empty()) // FIXME return; CCameraManager& rkCmrMgr = CCameraManager::Instance(); CCamera* pkCmrCur = rkCmrMgr.GetCurrentCamera(); if (pkCmrCur) { pkCmrCur->EndDrag(); } SetCursorNum(NORMAL); if (CURSOR_MODE_HARDWARE == GetCursorMode()) { SetCursorVisible(TRUE); } }
and
void CPythonApplication::DestroyCursors() { for (auto itor = m_CursorHandleMap.begin(); itor != m_CursorHandleMap.end(); ++itor) DestroyCursor((HCURSOR)itor->second); m_CursorHandleMap.clear(); // FIXME }
-
1
-
-
On 6/7/2020 at 12:45 PM, Vaynz said:
Search prGuildNameInstance->SetOutline(false); Replace prGuildNameInstance->SetOutline(false);
-
2
-
-
# update added 1.6 lib src
-
3
-
-
ChuCk core confirmed!
-
1
-
-
-
Spoiler
#ifndef __INC_METIN_II_STL_H__ #define __INC_METIN_II_STL_H__ #include <vector> #include <string> #include <map> #include <list> #include <functional> #include <stack> #include <set> #include <algorithm> #ifdef __GNUC__ #include <ext/functional> #endif #ifdef min #undef min #endif #ifdef max #undef max #endif #ifdef minmax #undef minmax #endif #ifndef itertype #define itertype(v) typeof((v).begin()) #endif inline void stl_lowers(std::string& rstRet) { for (size_t i = 0; i < rstRet.length(); ++i) rstRet[i] = tolower(rstRet[i]); } struct stringhash { size_t operator () (const std::string & str) const { const unsigned char * s = (const unsigned char*) str.c_str(); const unsigned char * end = s + str.size(); size_t h = 0; while (s < end) { h *= 16777619; h ^= *(s++); } return h; } }; // code from tr1/functional_hash.h template<typename T> struct hash; template<typename _Tp> struct hash<_Tp*> : public std::unary_function<_Tp*, std::size_t> { std::size_t operator()(_Tp* __p) const { return reinterpret_cast<std::size_t>(__p); } }; namespace std { template <class container, class Pred> void erase_if (container & a, typename container::iterator first, typename container::iterator past, Pred pred) { while (first != past) if (pred(*first)) a.erase(first++); else ++first; } template <class container> void wipe(container & a) { typename container::iterator first, past; first = a.begin(); past = a.end(); while (first != past) delete *(first++); a.clear(); } template <class container> void wipe_second(container & a) { typename container::iterator first, past; first = a.begin(); past = a.end(); while (first != past) { delete first->second; ++first; } a.clear(); } template <typename T> T min(T a, T b) { return a < b ? a : b; } template <typename T> T max(T a, T b) { return a > b ? a : b; } template <typename T> T minmax(T min, T value, T max) { T tv; tv = (min > value ? min : value); return (max < tv) ? max : tv; } template <class _Ty> class void_mem_fun_t : public unary_function<_Ty *, void> { public: explicit void_mem_fun_t(void (_Ty::*_Pm)()) : _Ptr(_Pm) { } void operator()(_Ty* p) const { ((p->*_Ptr)()); } private: void (_Ty::*_Ptr)(); }; template<class _Ty> inline void_mem_fun_t<_Ty> void_mem_fun(void (_Ty::*_Pm)()) { return (void_mem_fun_t<_Ty>(_Pm)); } template<class _Ty> class void_mem_fun_ref_t : public unary_function<_Ty, void> { public: explicit void_mem_fun_ref_t(void (_Ty::*_Pm)()) : _Ptr(_Pm) {} void operator()(_Ty& x) const { return ((x.*_Ptr)()); } private: void (_Ty::*_Ptr)(); }; template<class _Ty> inline void_mem_fun_ref_t<_Ty> void_mem_fun_ref(void (_Ty::*_Pm)()) { return (void_mem_fun_ref_t< _Ty>(_Pm)); } }; #endif
-
Can you show me what it contains ../../common/stl.h?
-
-
Because your client write wrong values for config when you close it.
-
No, move it to MSVC configuration or after endif(POLICY CMP0043)
-
1 minute ago, viperkit said:
Have you had this error?
Thanks for the answer, I'm trying to solve it!
Yes, if the platform selected is Win32 you must to define _USE_32BIT_TIME_T because time_t is by default __time64_t .
If you run in debug mode you can get a runtime error if isn't defined _USE_32BIT_TIME_T
That cmake project doesn't add definition for _USE_32BIT_TIME_T.
You can solve like this:
if(CMAKE_SIZEOF_VOID_P MATCHES 4) add_definitions(-D_USE_32BIT_TIME_T) endif()
Generate and rebuild.
-
1
-
-
-
If you have that starter I'm curious what's hiding inside, I can look.
Player Block System
in Features & Metin2 Systems
Posted
ez sql inject xd