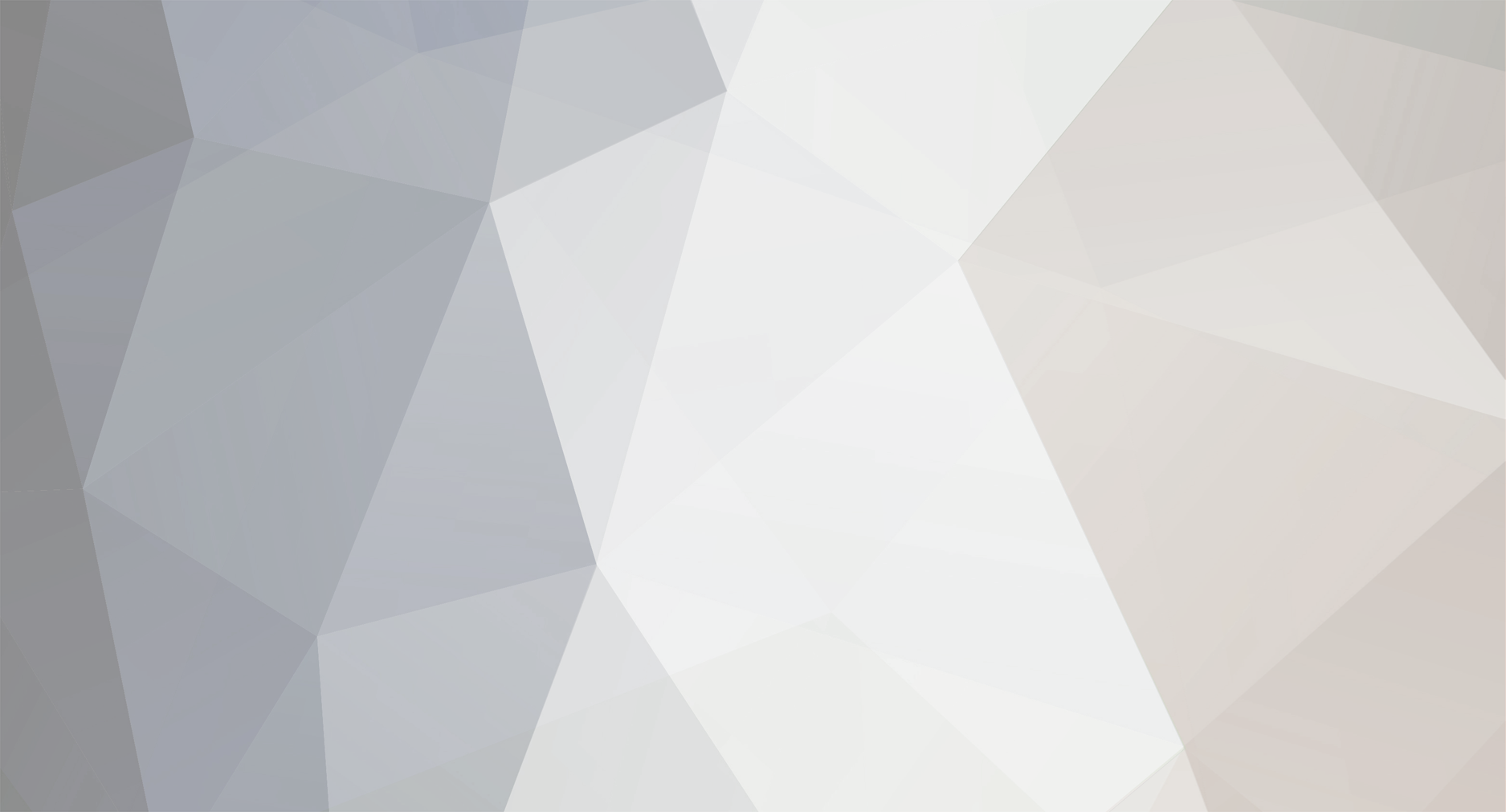
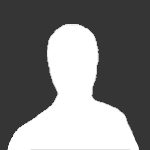
bnq-m
-
Posts
8 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Forums
Store
Third Party - Providers Directory
Feature Plan
Release Notes
Docs
Events
Posts posted by bnq-m
-
-
2 hours ago, Shogun said:
Find in the page the following:
SessionHandlerInterface
And paste here what comes after that.
Quote<?php
/**
* Created by PhpStorm.
* User: viperoo
* Date: 29.11.15
* Time: 19:48
*/
class DatabaseSession implements SessionHandlerInterface
{
protected $db;
protected $ip;
protected $ua;
protected $timeout;
protected $table = 'sessions';public function __construct($timeout = 216000)
{
if(admlt() || gmlt()) $timeout = 5184000;
$pdo = new PDO('mysql:host=host_ip;port=3306;dbname=site', 'elo', 'asdasd');$this->db = $pdo;
$this->table = 'sessions';
$this->timeout = $timeout;
}/**
* PHP >= 5.4.0<br/>
* Close the session
*
* @link http://php.net/manual/en/sessionhandlerinterafce.close.php
* @return bool <p>
* The return value (usually TRUE on success, FALSE on failure).
* Note this value is returned internally to PHP for processing.
* </p>
*/
public function close()
{
return true;
}/**
* PHP >= 5.4.0<br/>
* Destroy a session
*
* @link http://php.net/manual/en/sessionhandlerinterafce.destroy.php
* @param int $session_id The session ID being destroyed.
* @return bool <p>
* The return value (usually TRUE on success, FALSE on failure).
* Note this value is returned internally to PHP for processing.
* </p>
*/
public function destroy($session_id)
{
$this->db->query("DELETE FROM {$this->table} WHERE session_id = '$session_id'");
}/**
* PHP >= 5.4.0<br/>
* Cleanup old sessions
*
* @link http://php.net/manual/en/sessionhandlerinterafce.gc.php
* @param int $maxlifetime <p>
* Sessions that have not updated for
* the last maxlifetime seconds will be removed.
* </p>
* @return bool <p>
* The return value (usually TRUE on success, FALSE on failure).
* Note this value is returned internally to PHP for processing.
* </p>
*/
public function gc($maxlifetime)
{
$date = new \DateTime();$date->modify('-' . $maxlifetime . ' seconds');
$this->db->query("DELETE FROM {$this->table} WHERE " . '`session_last_activity` < \'' . $date->format("Y-m-d H:i:s") . '\'');
return true;
}/**
* PHP >= 5.4.0<br/>
* Initialize session
*
* @link http://php.net/manual/en/sessionhandlerinterafce.open.php
* @param string $save_path The path where to store/retrieve the session.
* @param string $session_id The session id.
* @return bool <p>
* The return value (usually TRUE on success, FALSE on failure).
* Note this value is returned internally to PHP for processing.
* </p>
*/
public function open($save_path, $session_id)
{
$this->gc($this->timeout);return true;
}/**
* PHP >= 5.4.0<br/>
* Read session data
*
* @link http://php.net/manual/en/sessionhandlerinterafce.read.php
* @param string $session_id The session id to read data for.
* @return string <p>
* Returns an encoded string of the read data.
* If nothing was read, it must return an empty string.
* Note this value is returned internally to PHP for processing.
* </p>
*/
public function read($session_id)
{
$data = $this->select("SELECT session_data FROM {$this->table} WHERE session_id = :id", array('id' => $session_id), true);return $data['session_data'];
}/**
* PHP >= 5.4.0<br/>
* Write session data
*
* @link http://php.net/manual/en/sessionhandlerinterafce.write.php
* @param string $session_id The session id.
* @param string $session_data <p>
* The encoded session data. This data is the
* result of the PHP internally encoding
* the $_SESSION superglobal to a serialized
* string and passing it as this parameter.
* Please note sessions use an alternative serialization method.
* </p>
* @return bool <p>
* The return value (usually TRUE on success, FALSE on failure).
* Note this value is returned internally to PHP for processing.
* </p>
*/
public function write($session_id, $session_data)
{if ($this->count("SELECT count(session_id) FROM {$this->table} WHERE session_id = :id", array('id' => $session_id)) > 0) {
$values = array(
'session_last_activity' => date("Y-m-d H:i:s", time()),
'session_data' => $session_data
);
$this->update($this->table, $values, '`session_id` = \'' . $session_id . '\' LIMIT 1');
} else {
$date = new \DateTime();
$values = array(
'session_id' => $session_id,
'session_user' => 0,
'session_last_activity' => $date->format("Y-m-d H:i:s"),
);$this->insert($this->table, $values);
}
}public function count($sql, $array = array())
{
$sth = $this->db->prepare($sql);
foreach ($array as $key => $value) {
$sth->bindValue("$key", $value);
}
$sth->execute();return $sth->fetchColumn();
}public function update($table, $data, $where)
{
ksort($data);$fieldDetails = null;
foreach ($data as $key => $value) {
$fieldDetails .= "`$key`=:$key,";
}
$fieldDetails = rtrim($fieldDetails, ',');$sth = $this->db->prepare("UPDATE $table SET $fieldDetails WHERE $where");
foreach ($data as $key => $value) {
$sth->bindValue(":$key", $value);
}$sth->execute();
}/**
* insert
*
* @param string $table A name of table to insert into
* @param array $data An associative array
* @return string
*/
public function insert($table, $data)
{
ksort($data);$fieldNames = implode('`, `', array_keys($data));
$fieldValues = ':' . implode(', :', array_keys($data));$sth = $this->db->prepare("INSERT INTO $table (`$fieldNames`) VALUES ($fieldValues)");
foreach ($data as $key => $value) {
$sth->bindValue(":$key", $value);
}$sth->execute();
return $this->db->lastInsertId();
}public function select($sql, $array = array(), $type = false, $fetchMode = \PDO::FETCH_ASSOC)
{
$sth = $this->db->prepare($sql);
if (is_array($array)) {
foreach ($array as $key => $value) {
$sth->bindValue("$key", $value);
}
}
$sth->execute();
if ($type == true) {
return $sth->fetch($fetchMode);
} else {
return $sth->fetchAll($fetchMode);
}
}
public function deleteUser($user)
{
$sth = $this->update($this->table, array('session_user'=>'0','session_data'=>NULL), '`session_user` = \'' . $user . '\' LIMIT 1');
return $sth;
}
public function setUser($user, $session_id)
{
$sth = $this->update($this->table, array('session_user'=>$user), '`session_id` = \'' . $session_id . '\' LIMIT 1');
return $sth;
}
public function getAllLogedSessions($min = 2)
{
$sth = $this->db->query("SELECT COUNT(*) FROM {$this->table} WHERE session_user != 0 AND session_last_activity >= DATE_SUB(NOW(), INTERVAL {$min} MINUTE)");
$row = $sth->fetch(PDO::FETCH_NUM);
return $row[0];
}
public function getAllSessions($min = 2)
{
$sth = $this->db->query("SELECT COUNT(*) FROM {$this->table} WHERE session_last_activity >= DATE_SUB(NOW(), INTERVAL {$min} MINUTE)");
$row = $sth->fetch(PDO::FETCH_NUM);
return $row[0];
}
public function getLastActivity($user)
{
$data = $this->select("SELECT session_last_activity FROM {$this->table} WHERE session_user = :id", array('id' => $user), true);return $data['session_last_activity'];
}
} -
7 hours ago, Shogun said:
I'll make a wild guess:
pkg add php74-session service apache24 restart
Quote
Updating FreeBSD repository catalogue...
FreeBSD repository is up to date.
All repositories are up to date.
Checking integrity... done (0 conflicting)
The most recent versions of packages are already installed
Already installed
-
5 hours ago, Shogun said:
So what's in /usr/local/www/apache24/data/inc/_config.php? I'm not psychic
Quote# ERRORs
ini_set('display_errors', 'on');
error_reporting(E_ALL & ~E_NOTICE & ~E_STRICT & ~E_DEPRECATED);# DEFLAUT TIME
date_default_timezone_set('Europe/Warsaw'); # Domyslne ustawienia czasu# OPTION DEBUG DATABASE
define('DB_DEBUG', true);define('CACHE_SITE', false);
define('CACHE_STYLES', false);
define('CACHE_SCRIPTS', false);
# CACHE TIMEs
define('CACHE_HTML_TIME', 15);# SITE PATH
define('DS', '/');
define('IMG', 'images' . DS);
define('MODULES', 'inc' . DS . 'modules' . DS);
define('GLOBALINC', 'includers' . DS);
define('LOCALE', 'locale' . DS);
define('USERPROFILE', 'profile' . DS);
define('GLOBALPAGES', 'pages' . DS);
define('DIRSUPPORT', 'support' . DS);define('ITEM_DESC_FILE', 'inc' . DS . 'client' . DS . 'itemdesc.txt');
define('ITEM_LIST_FILE', 'inc' . DS . 'client' . DS . 'item_list.txt');
define('ITEM_NAMES_FILE', 'inc' . DS . 'client' . DS . 'item_names.txt');
define('DIR_IMAGE_ITEMS', 'inc' . DS . 'client' . DS . 'item' . DS);define('ACP_FOLDER', 'admin' . DS);
# LOAD OTHER CONFIGs
require_once realpath($_SERVER['DOCUMENT_ROOT']) . DS . 'inc' . DS . '_setings' . DS . '_setings_admins.php';function __autoload($name)
{
include_once realpath($_SERVER['DOCUMENT_ROOT']) . DS . 'inc' . DS . 'class' . DS . $name . '.php';
}ini_set("session.gc_probability", 1);
ini_set("session.gc_divisor", 100);
$session = new DatabaseSession();
session_set_save_handler(
array($session, 'open'),
array($session, 'close'),
array($session, 'read'),
array($session, 'write'),
array($session, 'destroy'),
array($session, 'gc')
);# SESSIONs
if(!isset($_SESSION)) session_start();
Quoteif(!isset($_SESSION)) session_start();
This is line 80
-
Its working but
QuoteWarning: session_start(): Failed to read session data: user (path: ) in /usr/local/www/apache24/data/inc/_config.php on line 80
-
9 hours ago, Shogun said:
Wow, Apache. Feels like 2008 all over again.
In the shell type:
pkg add php74-pdo_mysql service apache24 restart
i have already installedQuotephp72-7.2.30 PHP Scripting Language
php72-bz2-7.2.30 The bz2 shared extension for php
php72-ctype-7.2.30 The ctype shared extension for php
php72-curl-7.2.30 The curl shared extension for php
php72-dom-7.2.30 The dom shared extension for php
php72-fileinfo-7.2.30 The fileinfo shared extension for php
php72-filter-7.2.30 The filter shared extension for php
php72-gd-7.2.30 The gd shared extension for php
php72-hash-7.2.30 The hash shared extension for php
php72-json-7.2.30 The json shared extension for php
php72-mbstring-7.2.30 The mbstring shared extension for php
php72-mysqli-7.2.30 The mysqli shared extension for php
php72-openssl-7.2.30 The openssl shared extension for php
php72-pdo-7.2.30 The pdo shared extension for php
php72-phar-7.2.30 The phar shared extension for php
php72-readline-7.2.30 The readline shared extension for php
php72-session-7.2.30 The session shared extension for php
php72-sockets-7.2.30 The sockets shared extension for php
php72-xml-7.2.30 The xml shared extension for php
php72-xmlreader-7.2.30 The xmlreader shared extension for php
php72-xmlwriter-7.2.30 The xmlwriter shared extension for php
php72-zip-7.2.30 The zip shared extension for php
php72-zlib-7.2.30 The zlib shared extension for php
-
hello, i have a problem with a page i downloaded from another forum
QuoteFatal error: Uncaught PDOException: could not find driver in /usr/local/www/apache24/data/inc/class/DatabaseSession.php:22 Stack trace: #0 /usr/local/www/apache24/data/inc/class/DatabaseSession.php(22): PDO->__construct('mysql:host=25.7...', 'site', 'password') #1 /usr/local/www/apache24/data/inc/_config.php(69): DatabaseSession->__construct() #2 /usr/local/www/apache24/data/BombWorkCMS.php(26): include('/usr/local/www/...') #3 {main} thrown in /usr/local/www/apache24/data/inc/class/DatabaseSession.php on line 22
FreeBSD 12.1 PHP 7.2
problem with site
in Community Support - Questions & Answers
Posted · Edited by bnq-m
after change