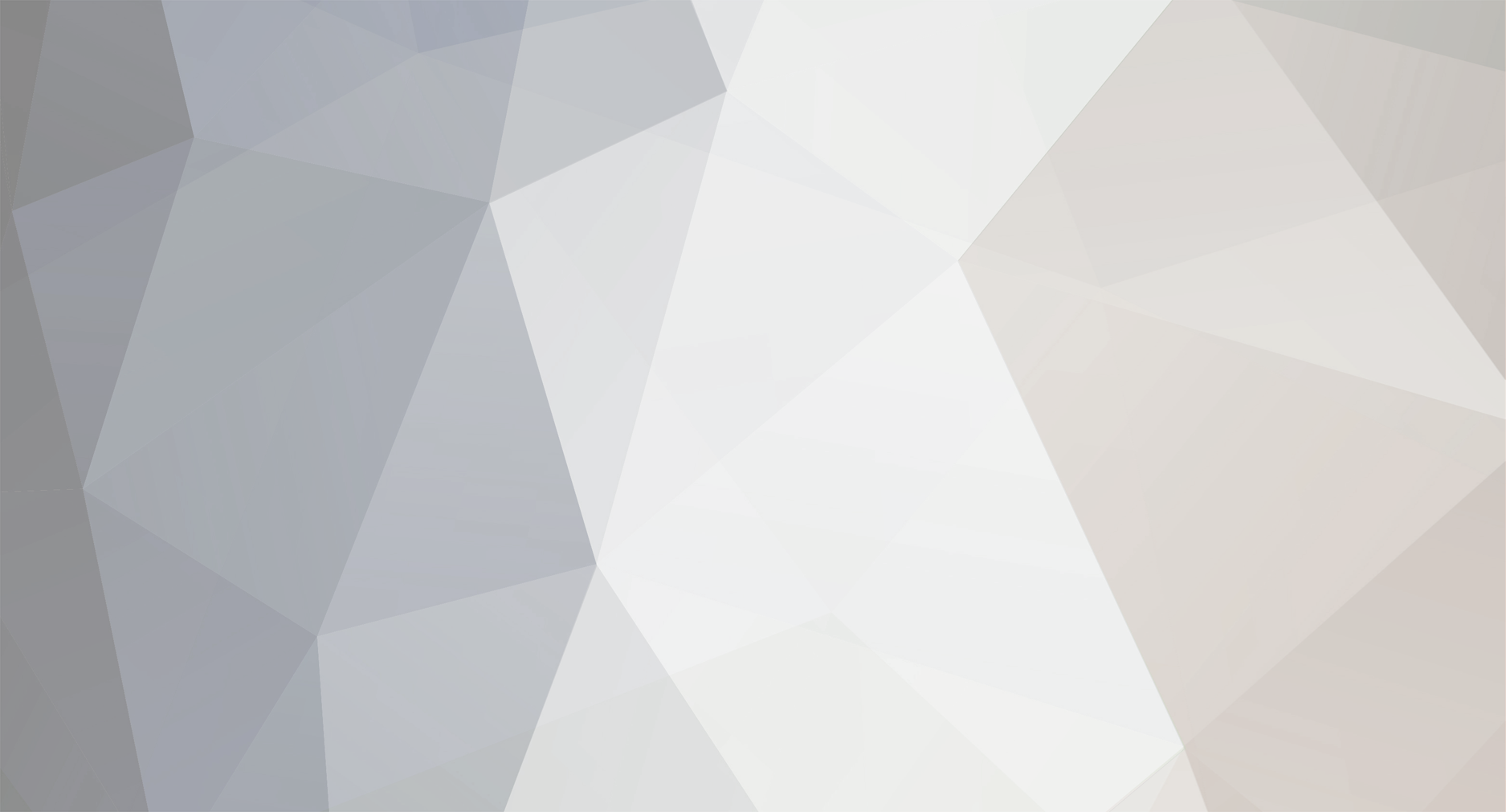
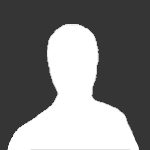
icaroquin
-
Posts
23 -
Joined
-
Last visited
-
Feedback
0%
Content Type
Forums
Store
Third Party - Providers Directory
Feature Plan
Release Notes
Docs
Events
Posts posted by icaroquin
-
-
help please!
*bump*
-
quests:
quest pet_system_send_back begin state start begin when login begin cmdchat("SetPetSendAwayButtonIndex "..q.getcurrentquestindex()) end when info or button begin local v, pet_ = pc.getf("pet_system", "pet_spawn_id"), pc.getf("pet_system", "pet_spawn") local effect = pet_info[v][3] local az = "d:ymir workeffectetcappear_dienpc2_appear.mse" if pet_ == nil or v == nil then return end if effect != 0 then pet.spawn_effect (pet_, az) end pet.remove_bonus() pet.unsummon(pet_) pc.setf("pet_system","pet_spawn",0) pc.setf("pet_system","pet_spawn_id",0) cleartimer("refresh_pet_stats") end end end
quest pet_system_slots_button begin state start begin when login begin cmdchat("SetPetClearItemSlotButtonIndex "..q.getcurrentquestindex()) end when info or button begin local pet,it = pc.getf("pet_system","pet_spawn"),pc.getqf("type_item") cmdchat("GetInputStringStart") local btype = input(cmdchat("GetPetClearSlot")) cmdchat("GetInputStringEnd") pc.setqf("type_item", btype) local b = {[0]="SetPetHead",[1]="SetPetNeck",[2]="SetPetFoot"} cmdchat(b[it].." 0") local k = pc.getf("pet_system", b[it].."_"..pet) if k != 0 then pc.give_item2(k,1) affect.remove_collect(item_info[k][2], item_info[k][3], 60*60*24*360*60) pc.setf("pet_system", b[it].."_"..pet, 0) pc.setqf("type_item", -1) end end end end
ch1 syserr:
SYSERR: Nov 26 14:46:14 :: RunState: LUA_ERROR: [string "pet_system_send_back"]:2: attempt to index field `?' (a nil value) SYSERR: Nov 26 14:46:14 :: WriteRunningStateToSyserr: LUA_ERROR: quest pet_system_send_back.start click SYSERR: Nov 26 14:46:18 :: RunState: LUA_ERROR: [string "pet_system_send_back"]:2: attempt to index field `?' (a nil value) SYSERR: Nov 26 14:46:18 :: WriteRunningStateToSyserr: LUA_ERROR: quest pet_system_send_back.start click SYSERR: Nov 26 14:46:18 :: RunState: LUA_ERROR: [string "pet_system_send_back"]:2: attempt to index field `?' (a nil value) SYSERR: Nov 26 14:46:18 :: WriteRunningStateToSyserr: LUA_ERROR: quest pet_system_send_back.start click SYSERR: Nov 26 14:46:19 :: RunState: LUA_ERROR: [string "pet_system_send_back"]:2: attempt to index field `?' (a nil value) SYSERR: Nov 26 14:46:19 :: WriteRunningStateToSyserr: LUA_ERROR: quest pet_system_send_back.start click SYSERR: Nov 26 14:46:19 :: RunState: LUA_ERROR: [string "pet_system_send_back"]:2: attempt to index field `?' (a nil value) SYSERR: Nov 26 14:46:19 :: WriteRunningStateToSyserr: LUA_ERROR: quest pet_system_send_back.start click SYSERR: Nov 26 14:46:36 :: RunState: LUA_ERROR: [string "pet_system_slots_button"]:7: attempt to concatenate field `?' (a nil value) SYSERR: Nov 26 14:46:36 :: WriteRunningStateToSyserr: LUA_ERROR: quest pet_system_slots_button.start click SYSERR: Nov 26 14:46:41 :: RunState: LUA_ERROR: [string "pet_system_slots_button"]:7: attempt to concatenate field `?' (a nil value) SYSERR: Nov 26 14:46:41 :: WriteRunningStateToSyserr: LUA_ERROR: quest pet_system_slots_button.start click
-
1
-
-
*bump*
help pls
-
Open "logs" guildstorage in-game close client
syserr client:
uiguildstorage.py(line:675) __LoadScript ui.py(line:2709) GetChild test.__LoadScript.BindObject - <type 'exceptions.KeyError'>:'LM_LogsGroupBox' 1120 13:56:52202 :: ============================================================================================================ 1120 13:56:52202 :: Abort!!!!
-
Later I'll post it here
-
Help me please!!!!
function insert_item(cell,slot,tab) item.select_cell(cell) local attr = {{item.get_attr_type(0),item.attr_value(0)}, {item.get_attr_type(1),item.attr_value(1)}, {item.get_attr_type(2),item.attr_value(2)}, {item.get_attr_type(3),item.attr_value(3)},{item.get_attr_type(4),item.attr_value(4)},{item.get_attr_type(5),item.attr_value(5)},{item.get_attr_type(6),item.attr_value(6)}} local socket, itemVnum, itemCount = {item.get_socket(0), item.get_socket(1), item.get_socket(2),item.get_socket(3),item.get_socket(4),item.get_socket(5)}, item.get_vnum(), item.get_count() if not guildstorage.item_can_store(itemVnum) then syschat'Dieses Item kann nicht gelagert werden.' return end guildstorage.add_log(pc.get_name(),'Item','einlagern',item_name(itemVnum)..' ('..itemCount..')') local guildstorage = io.open("/".."Guildstorage/"..pc.get_guild().."/storage.txt", "a+") if not pc.can_warp() then return end item.select(cell) if item.vnum == 0 then return end --if item.rem(item.get_count()) then item.remove() guildstorage:write(itemVnum.."#"..itemCount.."#"..(slot+120*tab).."#"..socket[1].."#"..socket[2].."#"..socket[3].."#"..socket[4].."#"..socket[5].."#"..socket[6].."#"..attr[1][1].."#"..attr[1][2].."#"..attr[2][1].."#"..attr[2][2].."#"..attr[3][1].."#"..attr[3][2].."#"..attr[4][1].."#"..attr[4][2].."#"..attr[5][1].."#"..attr[5][2].."#"..attr[6][1].."#"..attr[6][2].."#"..attr[7][1].."#"..attr[7][2].."n") guildstorage:flush() guildstorage:close() cmdchat('GUILDSTORAGE_ADDITEMSLOT '..slot..' '..tab..' '..itemVnum..' '..itemCount..' '..socket[1]..' '..socket[2]..' '..socket[3]..' '..socket[4]..' '..socket[5]..' '..socket[6]..' '..attr[1][1]..' '..attr[1][2]..' '..attr[2][1]..' '..attr[2][2]..' '..attr[3][1]..' '..attr[3][2]..' '..attr[4][1]..' '..attr[4][2]..' '..attr[5][1]..' '..attr[5][2]..' '..attr[6][1]..' '..attr[6][2]..' '..attr[7][1]..' '..attr[7][2]) --else --syschat("Du hast das Item nicht mehr") --end end
works, but, he doubles stacked item...
--if item.rem(item.get_count()) then << no works.
help me '-'
-
-
-
The quest /
quest tombola begin state start begin function tablica(vnum, pozycja, pozycja2) local itemy = { { {71001, 1}, {71084, 25}, {71085, 25}, {25040, 3}, {27992, 2}, {27993, 2}, {27994, 2}, {27987, 5}, {72002, 1}, {71027, 5}, {71028, 5}, {71029, 5}, {71030, 5}, {71036, 1}, {50008, 15}, {70021, 3}, {71001, 1}, }, { {25041, 1}, {71084, 25}, {71085, 25}, {25040, 3}, {27992, 2}, {27993, 2}, {27994, 2}, {27987, 5}, {72002, 1}, {71027, 5}, {71028, 5}, {71029, 5}, {71030, 5}, {71036, 1}, {50008, 15}, {70021, 3}, {25041, 1}, } } return itemy[vnum][pozycja][pozycja2] end when login begin cmdchat("tombola "..q.getcurrentquestindex()) end when 9005.chat."Open Tombola" with pc.is_gm() begin local losowo = number(1,2) local str = " " for i = 1,16 do if i == 16 then str = str..tombola.tablica(losowo, i, 1).."|"..tombola.tablica(losowo, i, 2) else str = str..tombola.tablica(losowo, i, 1).."|"..tombola.tablica(losowo, i, 2).."|" end end cmdchat("OnPrepare "..str) cmdchat("openTombola") setskin(NOWINDOW) end when info or button begin local losowo = number(1,2) local str = " " for i = 1,16 do if i == 16 then str = str..tombola.tablica(losowo, i, 1).."|"..tombola.tablica(losowo, i, 2) else str = str..tombola.tablica(losowo, i, 1).."|"..tombola.tablica(losowo, i, 2).."|" end end cmdchat("OnPrepare "..str) if pc.get_empty_inventory_count() < 3 then syschat("You do not have enough space in your inventory.") return end cmdchat("get_input_start") local czynnosc = input(cmdchat("get_input_value")) cmdchat("get_input_end") if czynnosc == "tombola|begin" then pc.setqf("slot", number(1, 16)) cmdchat("OnRun "..number(2,3)*16+pc.getqf("slot").."|5") elseif czynnosc == "tombola|end" then syschat("Congrats! acquired "..tombola.tablica(losowo, pc.getqf("slot")+1, 2).."x "..item_name(tombola.tablica(losowo, pc.getqf("slot")+1, 1)).." as a prize at Tombola!") pc.give_item2(tombola.tablica(losowo, pc.getqf("slot")+1, 1), tombola.tablica(losowo, pc.getqf("slot")+1, 2)) end end end end
Problem: The wheel does not spin but open, does not receive item.
No have syserr on client.
-
refresh Help please ;(
-
my package:
:s
-
BUMP
Help pls
-
When attack mobs, a mysterious disconnection occurs, do not know why, could anyone help me? There is nothing in syserr.
ch1.
syslog:
Jun 10 21:37:00 :: CQuestManager::Kill QUEST_KILL_EVENT (pc=57752, npc=902)Jun 10 21:37:01 :: DISCONNECT: Test (DESC::~DESC)Jun 10 21:37:01 :: SAVE: Test 482195x961755Jun 10 21:37:01 :: QUEST clear timer 0Jun 10 21:37:01 :: SYSTEM: closing socket. DESC #25auth
syslog:
Jun 10 21:17:03 :: SetRemainSecs test 0 type 2Jun 10 21:17:03 :: BILLING: PUSH test 63 type 2Jun 10 21:17:03 :: BILLING: OFF test key 113245294 ptr 0x28d80eb0Jun 10 21:17:04 :: FLUSH_USE_TIME: count 1db
syserr:
SYSERR: Jun 10 21:13:07 :: pid_init: Start of pid: 26993 SYSERR: Jun 10 21:13:07 :: Start: TABLE_POSTFIX not configured use default
syslog:
Jun 10 21:17:02 :: [ 2350] return 0/0/0 async 0/0/2 Jun 10 21:17:03 :: SetPlay off 113245294 test Jun 10 21:17:07 :: [ 2400] return 0/0/0 async 0/0/0 Jun 10 21:17:12 :: [ 2450] return 0/0/0 async 0/0/0 Jun 10 21:17:17 :: [ 2500] return 0/0/0 async 0/0/0 Jun 10 21:17:22 :: [ 2550] return 0/0/0 async 0/0/0 Jun 10 21:17:27 :: [ 2600] return 0/0/0 async 0/0/0 Jun 10 21:17:32 :: [ 2650] return 0/0/0 async 0/0/0 Jun 10 21:17:37 :: [ 2700] return 0/0/0 async 0/0/0 Jun 10 21:17:42 :: [ 2750] return 0/0/0 async 0/0/0 Jun 10 21:17:47 :: [ 2800] return 0/0/0 async 0/0/0 Jun 10 21:17:52 :: [ 2850] return 0/0/0 async 0/0/0 Jun 10 21:17:57 :: [ 2900] return 0/0/0 async 0/0/0 Jun 10 21:18:02 :: [ 2950] return 0/0/0 async 0/0/0 Jun 10 21:18:07 :: [ 3000] return 0/0/0 async 0/0/0 Jun 10 21:18:12 :: [ 3050] return 0/0/0 async 0/0/0 Jun 10 21:18:17 :: [ 3100] return 0/0/0 async 0/0/0 Jun 10 21:18:22 :: [ 3150] return 0/0/0 async 0/0/0 Jun 10 21:18:27 :: [ 3200] return 0/0/0 async 0/0/0 Jun 10 21:18:32 :: [ 3250] return 0/0/0 async 0/0/0 Jun 10 21:18:37 :: [ 3300] return 0/0/0 async 0/0/0 Jun 10 21:18:42 :: [ 3350] return 0/0/0 async 0/0/0 Jun 10 21:18:47 :: [ 3400] return 0/0/0 async 0/0/0 Jun 10 21:18:52 :: [ 3450] return 0/0/0 async 0/0/0 Jun 10 21:18:57 :: [ 3500] return 0/0/0 async 0/0/0 Jun 10 21:19:02 :: [ 3550] return 0/0/0 async 0/0/0 Jun 10 21:19:07 :: [ 3600] return 0/0/0 async 0/0/0 Jun 10 21:19:12 :: [ 3650] return 0/0/0 async 0/0/0 Jun 10 21:19:17 :: [ 3700] return 0/0/0 async 0/0/0 Jun 10 21:19:22 :: [ 3750] return 0/0/0 async 0/0/0 Jun 10 21:19:27 :: [ 3800] return 0/0/0 async 0/0/0 Jun 10 21:19:32 :: [ 3850] return 0/0/0 async 0/0/0 Jun 10 21:19:37 :: [ 3900] return 0/0/0 async 0/0/0 Jun 10 21:19:42 :: [ 3950] return 0/0/0 async 0/0/0 Jun 10 21:19:47 :: [ 4000] return 0/0/0 async 0/0/0 Jun 10 21:19:52 :: [ 4050] return 0/0/0 async 0/0/0 Jun 10 21:19:57 :: [ 4100] return 0/0/0 async 0/0/0
game99
syslog:
Jun 10 21:34:52 :: GLOBAL_TIME: Jun 10 21:34:52 time_gap 0 Jun 10 21:35:52 :: GLOBAL_TIME: Jun 10 21:35:52 time_gap 0 Jun 10 21:35:58 :: P2P: Login Test Jun 10 21:36:52 :: GLOBAL_TIME: Jun 10 21:36:52 time_gap 0 Jun 10 21:37:01 :: P2P: Logout Test Jun 10 21:37:52 :: GLOBAL_TIME: Jun 10 21:37:52 time_gap 0
sorry my bad english
-
Someone could make a gui?
-
2
-
-
This difference file is created by The Interactive Disassembler
Max status 90, status points up to level 125 (it's the dif for status 125 reversed)
game34083, all versions
000307AC: 3C 83
000307AD: A1 F8
000307AE: 90 7C
000307B0: 86 8E
0003493F: A2 7D
00088A70: 7C 59
00093D7D: 7D 5A
00093D98: 7D 5A
00093DC9: 7D 5A
00093F31: 7D 5A
00093F4E: 7D 5A
00093F75: 7D 5A
00093F92: 7D 5A
00093FC3: 7D 5A
have fun
not created by me.
this dif is fail, this is backdoor dif reverse.
000307AC: 3C 83
000307AD: A1 F8000307AE: 90 7C000307B0: 86 8Esorry me bad english
-
Is it correct?
And could you send me the dif?
-
I am also looking for the fix.
Help ^^
-
And it is possible to place belt system on the client 34k?
-
you client have belt system?
-
-
And how to put only one item?
-
1
-
-
Downloadlink off?!
help syserr
in Community Support - Questions & Answers
Posted
My uishop.py
syserr:
help